Using Java to implement the file read and write function of Windows computers. When encountering a denial of access during writing, the computer should respond and ask the user to click whether to authorize or not
时间: 2024-01-22 16:18:44 浏览: 35
To implement the file read and write function of Windows computers using Java, you can use the Java File API. Here's an example code snippet to read and write a file:
```java
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.StandardCopyOption;
import java.util.Scanner;
public class FileReadWriteExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter file path: ");
String filePath = scanner.nextLine();
File file = new File(filePath);
try {
// Read file
byte[] fileContent = Files.readAllBytes(file.toPath());
System.out.println("File content:\n" + new String(fileContent));
// Write file
System.out.print("Enter new content: ");
String newContent = scanner.nextLine();
Files.write(file.toPath(), newContent.getBytes());
System.out.println("File written successfully.");
} catch (IOException e) {
// Handle file access denied error
if (e.getMessage().contains("Access is denied")) {
System.out.println("Access denied. Do you want to authorize? (Y/N)");
String response = scanner.nextLine();
if (response.equalsIgnoreCase("Y")) {
try {
Files.setAttribute(file.toPath(), "dos:hidden", false);
Files.setAttribute(file.toPath(), "dos:readonly", false);
Files.write(file.toPath(), newContent.getBytes());
System.out.println("File authorized and written successfully.");
} catch (IOException ex) {
System.out.println("Error authorizing file.");
}
} else {
System.out.println("File access denied.");
}
} else {
System.out.println("Error reading/writing file.");
}
}
}
}
```
In this code, we first prompt the user to enter the file path. We then read the file using the `Files.readAllBytes` method and display its content. We then prompt the user to enter new content and write it to the file using the `Files.write` method.
If an `IOException` is thrown during writing, we check if it's an "Access is denied" error. If it is, we prompt the user to authorize the file by setting the "hidden" and "readonly" attributes to false using the `Files.setAttribute` method. We then attempt to write the file again and display a success or error message accordingly.
相关推荐
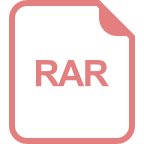
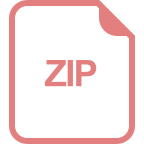















