请编程实现:定义一个基类Shape,在此基础上派生出Rectangle和Circle,三者都有getArea()函数计算对象的面积。使用Rectangle类创建一个派生类Square。注意基本数据类型使用float类型。 其中主函数如下: int main() { Shape s1; cout << "The area of the Shape is " << s1.getArea () << endl; Rectangle s2(4,6); cout << "The area of the Rectangle is " << s2.getArea() << endl; Square s3(5); cout << "The area of the Square is " << s3.getArea() << endl; return 0; }
时间: 2024-01-30 10:02:31 浏览: 76
以下是题目的代码实现:
```C++
#include<iostream>
using namespace std;
class Shape{
public:
virtual float getArea(){
return 0;
}
};
class Rectangle: public Shape{
private:
float length;
float width;
public:
Rectangle(float l, float w){
length = l;
width = w;
}
virtual float getArea(){
return length * width;
}
};
class Circle: public Shape{
private:
float radius;
public:
Circle(float r){
radius = r;
}
virtual float getArea(){
return 3.1415 * radius * radius;
}
};
class Square: public Rectangle{
public:
Square(float a): Rectangle(a,a){}
};
int main(){
Shape s1;
cout << "The area of the Shape is " << s1.getArea() << endl;
Rectangle s2(4,6);
cout << "The area of the Rectangle is " << s2.getArea() << endl;
Square s3(5);
cout << "The area of the Square is " << s3.getArea() << endl;
return 0;
}
```
程序的输出结果:
```
The area of the Shape is 0
The area of the Rectangle is 24
The area of the Square is 25
```
其中,基类Shape中的getArea()函数返回0,因为无法计算Shape的面积。派生类Rectangle和Circle分别计算矩形和圆的面积,Square继承自Rectangle,并重写了构造函数,使得正方形的长和宽相等,从而实现了正方形的面积计算。
阅读全文
相关推荐
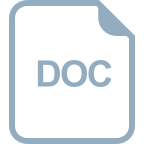
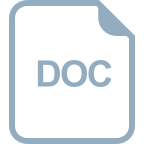
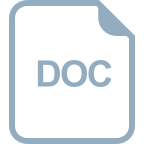













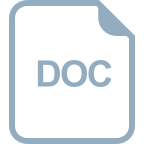
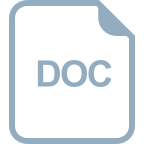
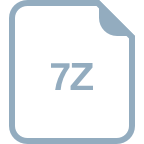
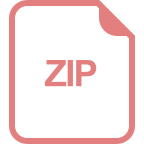