int compareTotalScore(const void *a, const void *b) { return (((Student *) b)->total_score - ((Student *) a)->total_score); } int compareCScore(const void *a, const void *b) { return (((Student *) b)->c_score - ((Student *) a)->c_score); }之后的代码是什么
时间: 2023-12-18 16:04:33 浏览: 100
根据你提供的代码片段,我可以给出一个使用这两个比较函数进行排序的示例代码。假设你有一个包含了多个学生信息的数组 `students`,每个学生都有一个 `total_score` 和 `c_score` 字段。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int total_score;
int c_score;
} Student;
int compareTotalScore(const void *a, const void *b) {
return (((Student *) b)->total_score - ((Student *) a)->total_score);
}
int compareCScore(const void *a, const void *b) {
return (((Student *) b)->c_score - ((Student *) a)->c_score);
}
int main() {
// 假设有三个学生
Student students[3];
// 初始化学生信息
students[0].total_score = 90;
students[0].c_score = 80;
students[1].total_score = 85;
students[1].c_score = 95;
students[2].total_score = 95;
students[2].c_score = 90;
// 使用 compareTotalScore 函数进行按总分降序排序
qsort(students, 3, sizeof(Student), compareTotalScore);
// 输出按总分排序后的结果
printf("按总分降序排序结果:\n");
for (int i = 0; i < 3; i++) {
printf("总分:%d, C语言成绩:%d\n", students[i].total_score, students[i].c_score);
}
// 使用 compareCScore 函数进行按C语言成绩降序排序
qsort(students, 3, sizeof(Student), compareCScore);
// 输出按C语言成绩排序后的结果
printf("\n按C语言成绩降序排序结果:\n");
for (int i = 0; i < 3; i++) {
printf("总分:%d, C语言成绩:%d\n", students[i].total_score, students[i].c_score);
}
return 0;
}
```
以上代码会输出两次排序后的结果:按总分降序排序和按C语言成绩降序排序。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
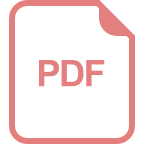
















