帮我创建一个无人机3D模型可旋转的QT类
时间: 2023-05-26 22:07:40 浏览: 228
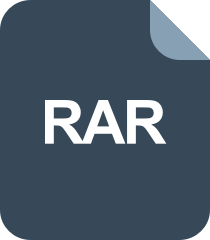
三叉戟无人机 3d模型(可商用)
以下是一个简单的无人机3D模型旋转的QT类示例:
```C++
#include <Qt3DExtras/QCylinderMesh>
#include <Qt3DExtras/QDiffuseMapMaterial>
#include <Qt3DExtras/QPhongAlphaMaterial>
#include <Qt3DExtras/QPhongMaterial>
#include <Qt3DExtras/QSphereMesh>
#include <Qt3DExtras/QTransform>
#include <Qt3DExtras/Qt3DWindow>
#include <Qt3DRender/QCamera>
#include <Qt3DRender/QPointLight>
#include <QMouseEvent>
class DroneModel : public QObject
{
Q_OBJECT
public:
explicit DroneModel(Qt3DCore::QEntity *parent = nullptr)
: m_rootEntity(new Qt3DCore::QEntity(parent))
{
createBody();
createBlades();
}
void setTranslation(const QVector3D &pos)
{
m_rootEntity->transform()->setTranslation(pos);
}
void setRotation(float angle, const QVector3D &axis)
{
m_rootEntity->transform()->setRotation(QQuaternion::fromAxisAndAngle(axis, angle));
}
Qt3DCore::QEntity *rootEntity() const
{
return m_rootEntity;
}
private:
void createBody()
{
static const float radius = 0.5f;
static const float height = 0.2f;
auto *bodyEntity = new Qt3DCore::QEntity(m_rootEntity);
auto *cylinderMesh = new Qt3DExtras::QCylinderMesh(bodyEntity);
cylinderMesh->setRadius(radius);
cylinderMesh->setLength(height * 3);
auto *material = new Qt3DExtras::QPhongMaterial(bodyEntity);
material->setDiffuse(QColor(128, 128, 128));
auto *transform = new Qt3DCore::QTransform(bodyEntity);
transform->setTranslation(QVector3D(0, 0, -height));
bodyEntity->addComponent(cylinderMesh);
bodyEntity->addComponent(material);
bodyEntity->addComponent(transform);
}
void createBlades()
{
static const float radius = 0.03f;
static const float height = 0.2f;
auto *bladeEntity = new Qt3DCore::QEntity(m_rootEntity);
auto *sphereMesh = new Qt3DExtras::QSphereMesh(bladeEntity);
sphereMesh->setRadius(radius * 2);
auto *material = new Qt3DExtras::QPhongAlphaMaterial(bladeEntity);
material->setAlpha(0.5f);
material->setDiffuse(QColor(255, 255, 255));
for (int i = 0; i < 4; ++i) {
auto *blade = new Qt3DCore::QEntity(bladeEntity);
auto *cylinderMesh = new Qt3DExtras::QCylinderMesh(blade);
cylinderMesh->setRadius(radius);
cylinderMesh->setLength(height);
auto *bladeTransform = new Qt3DCore::QTransform(blade);
bladeTransform->setRotation(QQuaternion::fromAxisAndAngle(QVector3D(0, 0, 1), i * 90));
auto *bladeMaterial = new Qt3DExtras::QPhongMaterial(blade);
bladeMaterial->setDiffuse(QColor(0, 255, 0)); // Set blade color
blade->addComponent(cylinderMesh);
blade->addComponent(bladeMaterial);
blade->addComponent(bladeTransform);
auto *sphere = new Qt3DCore::QEntity(blade);
sphere->addComponent(sphereMesh);
sphere->addComponent(material);
}
}
private:
Qt3DCore::QEntity *m_rootEntity;
};
class DroneViewer : public Qt3DExtras::Qt3DWindow
{
Q_OBJECT
public:
explicit DroneViewer(QWidget *parent = nullptr)
: Qt3DExtras::Qt3DWindow(parent)
, m_drone(new DroneModel(rootEntity()))
{
defaultCamera()->setNearPlane(0.1f);
defaultCamera()->setFarPlane(100.0f);
defaultCamera()->setFieldOfView(45.0f);
defaultCamera()->setPosition(QVector3D(0, 4, 4));
defaultCamera()->setViewCenter(QVector3D(0, 0, 0));
auto *lightEntity = new Qt3DCore::QEntity(rootEntity());
auto *light = new Qt3DRender::QPointLight(lightEntity);
light->setColor(Qt::white);
light->setIntensity(1);
light->setConstantAttenuation(1);
light->setLinearAttenuation(0);
light->setQuadraticAttenuation(0);
auto *lightTransform = new Qt3DCore::QTransform(lightEntity);
lightTransform->setTranslation(QVector3D(5, 5, 5));
lightEntity->addComponent(light);
lightEntity->addComponent(lightTransform);
setRootEntity(rootEntity());
}
protected:
void mousePressEvent(QMouseEvent *e) override
{
m_lastPos = e->pos();
}
void mouseMoveEvent(QMouseEvent *e) override
{
Qt::MouseButtons buttons = e->buttons();
if (!(buttons & Qt::LeftButton)) {
return;
}
QPoint delta = e->pos() - m_lastPos;
QVector3D axis = QVector3D(delta.y(), delta.x(), 0.0f).normalized();
float angle = delta.manhattanLength() / 10.0f;
m_drone->setRotation(m_drone->rootEntity()->transform()->rotation().angle() + angle, axis);
m_lastPos = e->pos();
}
private:
DroneModel *m_drone;
QPoint m_lastPos;
};
```
您可以将上述代码用作您自己的QT项目中的QT文件,然后在视图中使用`DroneViewer`类即可旋转无人机3D模型。
阅读全文
相关推荐
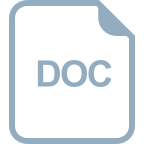
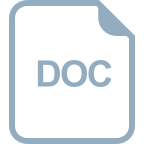

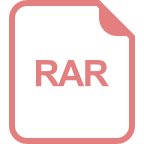
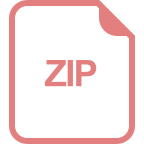
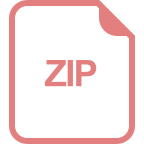
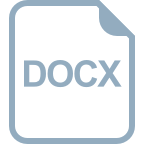
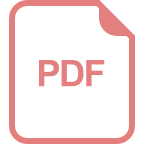
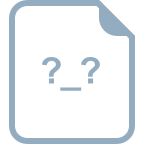