#include <iostream> #include <graphics.h> int main() { // 初始化图形库 initgraph(640, 480); // 绘制正方形 setcolor(YELLOW); setfillstyle(SOLID_FILL, YELLOW); rectangle(320, 240, 340, 260); // 关闭图形库 closegraph(); return 0; }改错
时间: 2024-01-10 18:04:15 浏览: 115
代码中似乎没有错别字或语法错误,但缺少了头文件的声明。正确的代码应该是:
```
#include <graphics.h>
#include <iostream>
int main() {
// 初始化图形库
initgraph(640, 480);
// 绘制正方形
setcolor(YELLOW);
setfillstyle(SOLID_FILL, YELLOW);
rectangle(320, 240, 340, 260);
// 关闭图形库
closegraph();
return 0;
}
```
需要注意的是,在使用 C++ 的 `iostream` 头文件时,我们通常习惯把它放在前面。但在这个程序中,由于需要使用 `graphics.h` 头文件中的函数,所以这个头文件需要先声明。
相关问题
#include <graphics.h> #include<easyx.h> #include<conio.h> #include<cmath> #include<iostream> //using namespace std; int main() { initgraph(600, 800); IMAGE img; loadimage(&img, _T("C:/Users/86181/Pictures/Camera Roll/imgBG.jpg")); Resize(NULL,img.getwidth(),img.getheight()); /* setbkcolor(RED); cleardevice();*/ putimage(0, 0, &img); setbkmode(RED); settextcolor(0xff0000); settextstyle(20, 0, _T("楷书")); _TCHAR topic[] = _T("送元二使安西"); _TCHAR poem[][20] = { _T("渭城朝雨浥轻尘,"),_T("客舍青青柳色新。"), _T("劝君更尽一杯酒,"),_T("西出阳关无故人。") }; int nHeight = textheight(topic[0]); outtextxy(30, 100, topic); for (int i = 0; i < 4; ++i) { outtextxy(20, 100 + nHeight * 1.5 * (2 + i), poem[i]); } _getch(); // 等待按键退出 closegraph(); // 关闭图形窗口 return 0; }
这段代码使用了C++的图形库来绘制图形界面。首先,通过`initgraph`函数初始化图形窗口的大小为600x800像素。然后,使用`loadimage`函数加载一张图片作为背景,并使用`putimage`函数将图片绘制到窗口中。接下来,设置文字的背景颜色为红色,文字颜色为蓝色,并设置文字的样式和大小。然后,使用`outtextxy`函数在窗口中输出一段文字。最后,使用`_getch`函数等待按键输入,以及`closegraph`函数关闭图形窗口。整个程序的功能是绘制一个带有背景图片和文字的图形界面。
#include<graphics.h> #include<conio.h> #include<iostream> using namespace std; class CObject { public: virtual void Draw() = 0; virtual void Update(float t) = 0; }; class Cball :public CObject { private: float m_x; float m_y; int m_r; int m_color; float m_Vy; public: Cball(float x, float y, int r, int color) { m_x = x; m_y = y; m_r = r; m_color = color; m_Vy = 0; } void Draw() { COLORREF color = getfillcolor(); setfillcolor(m_color); solidcircle(m_x, m_y, m_r); } void Update(float t) { m_Vy += -9.8 * t; m_y += m_Vy * t + 0.5 * (-9.8) * t * t; if (m_y < m_r - 200) { m_Vy = -0.8 * m_Vy; m_y = m_r - 200; } } }; int main() { initgraph(800, 600); setorigin(400, 300); setaspectratio(1, -1); cleardevice(); static const COLORREF groundColor = RGB(100, 100, 100); // 绘制地面直线 line(-400, -180, 400, -180); // 绘制地面斜线 for (int i = -400; i < 400; i += 10) { // 绘制斜线 line(i,-181 , i,-179 ); } // 设置地面颜色 setcolor(groundColor); Cball ball(20, 200, 20, RED); //BeginBatchDraw(); for (int i = 0; i < 1000; ++i) { BeginBatchDraw(); cleardevice(); ball.Draw(); ball.Update(0.05); Sleep(30); EndBatchDraw(); } //EndBatchDraw(); _getch(); closegraph(); return 0; }为什么我的代码运行后不出现大地,只出现小球运动呢
你的代码中绘制了地面直线和斜线,但是没有设置填充颜色。所以即使绘制了这线段,也无法看到地面效果。你可以在设置地面颜色之后,添加以下代码来填充地面:
```cpp
// 填充地面颜色
bar(-400, -180, 400, -179);
```
这样就能够看到地面了。另外,你还需要确保你的绘图环境正常初始化和关闭,在使用 `initgraph()` 初始化绘图窗口之前,确保你已经正确安装了 EasyX 图形库,并且头文件和库文件的路径设置正确。
阅读全文
相关推荐
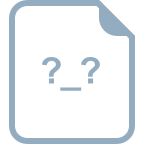
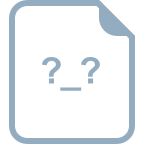
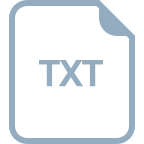
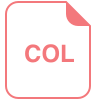













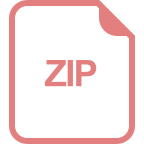