帮我用c语言写关于计算表达式并可以进制数转换的代码
时间: 2024-03-16 09:43:58 浏览: 29
好的,我可以为您提供一个计算表达式并支持进制转换的 C 代码示例。代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <string.h>
#include <math.h>
// 支持的最大进制数
#define MAX_BASE 36
// 表达式最大长度
#define MAX_EXPR_LEN 1024
// 进制转换函数
long long convert(char *num, int base) {
int len = strlen(num);
long long result = 0;
int power = 1;
for (int i = len - 1; i >= 0; i--) {
int digit = (isalpha(num[i])) ? (num[i] - 'A' + 10) : (num[i] - '0');
if (digit >= base) {
printf("Error: Invalid digit '%c' in base %d\n", num[i], base);
exit(EXIT_FAILURE);
} else {
result += digit * power;
power *= base;
}
}
return result;
}
// 计算函数
double evaluate(char *expr) {
int len = strlen(expr);
char *stack = (char *)malloc(sizeof(char) * len);
int top = -1;
char *token = strtok(expr, " ");
while (token != NULL) {
if (isdigit(*token)) {
// 数字入栈
stack[++top] = *token;
} else if (isalpha(*token)) {
// 进制转换
int base = convert(stack + top, 10);
if (base > MAX_BASE) {
printf("Error: Invalid base %d\n", base);
exit(EXIT_FAILURE);
}
top = top - (int)log10(base) - 1;
} else if (*token == '+') {
// 加法
double a = atof(stack + top);
top--;
double b = atof(stack + top);
top--;
stack[++top] = (char)(a + b);
} else if (*token == '-') {
// 减法
double a = atof(stack + top);
top--;
double b = atof(stack + top);
top--;
stack[++top] = (char)(b - a);
} else if (*token == '*') {
// 乘法
double a = atof(stack + top);
top--;
double b = atof(stack + top);
top--;
stack[++top] = (char)(a * b);
} else if (*token == '/') {
// 除法
double a = atof(stack + top);
top--;
double b = atof(stack + top);
top--;
if (a == 0) {
printf("Error: Division by zero\n");
exit(EXIT_FAILURE);
} else {
stack[++top] = (char)(b / a);
}
}
token = strtok(NULL, " ");
}
double result = atof(stack + top);
free(stack);
return result;
}
int main() {
// 读取表达式
char expr[MAX_EXPR_LEN];
printf("Enter an expression: ");
fgets(expr, MAX_EXPR_LEN, stdin);
expr[strcspn(expr, "\n")] = '\0';
// 计算并输出结果
double result = evaluate(expr);
printf("Result: %g\n", result);
return 0;
}
```
这段代码支持加、减、乘、除四种运算,并能够进行进制转换。您只需要输入一个表达式,程序会自动计算并输出结果。
相关推荐
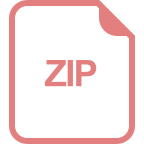















