已知一个单链表中的数据元素含有三类字符(即字母字符,数字字符和其它字符),试编写算法,构造三个循环链表,使每个循环链表中只含有同一类的字符,且利用原表中的结点空间作为这三个表的结点空间。
时间: 2023-05-02 15:01:52 浏览: 144
这段文本描述了如何构建一个单链表,并且每个节点包含三类字符(即字母字符、数字字符以及其他字符)。编写算法,构造三个循环链表,使每个循环链表中只含有同一类字符,并利用原表中的结点空间作为这三个表的结点空间。
相关问题
已知由不具有头结点的单链表表示的线性表中,含有三类字符的数据元素(字母、数字和其他字符),试编写算法构造三个以循环链表表示的线性表,使每个表中只含有同一类的字符,且利用原表中的结点空间,头结点可另辟空
间。
算法思路:
1. 定义三个循环链表,分别表示字母、数字和其他字符。
2. 遍历原链表,将每个结点根据其数据元素类型插入到对应的循环链表中。
3. 在原链表的头部插入一个新结点作为头结点,使原链表成为带头结点的单链表。
4. 返回三个循环链表的头结点。
算法实现:
```
typedef struct node {
char data;
struct node *next;
} Node;
Node *createCircularList() {
Node *head = (Node *)malloc(sizeof(Node));
head->next = head;
return head;
}
void insertNode(Node *head, char data) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
newNode->next = head->next;
head->next = newNode;
}
Node *separateList(Node *list) {
Node *letterList = createCircularList();
Node *digitList = createCircularList();
Node *otherList = createCircularList();
Node *p = list->next;
while (p != list) {
if (isalpha(p->data)) {
insertNode(letterList, p->data);
} else if (isdigit(p->data)) {
insertNode(digitList, p->data);
} else {
insertNode(otherList, p->data);
}
p = p->next;
}
list->data = 'H';
list->next = letterList;
letterList->data = 'A';
letterList->next = digitList;
digitList->data = '';
digitList->next = otherList;
otherList->data = '#';
otherList->next = list;
return letterList;
}
```
算法说明:
1. createCircularList() 函数用于创建一个空的循环链表,返回头结点指针。
2. insertNode() 函数用于在循环链表的头部插入一个新结点,数据元素为 data。
3. separateList() 函数用于将原链表中的结点按照数据元素类型分别插入到三个循环链表中,并将原链表转化为带头结点的单链表。
4. 在返回循环链表头结点之前,需要将原链表的头结点指向循环链表的第一个结点,以便于后续操作。
5. 时间复杂度为 O(n),空间复杂度为 O(1)。
已知由一个线性链表表示的线性表中含有三类字符的数据元素(如:字母字符、数字字符和其他字符),试编写算法将该线性表分割为三个循环链表,其中每个循环链表表示的线性表中均只含一类字符。
可以按照以下步骤编写算法将线性链表分割为三个循环链表,其中每个循环链表只含一类字符:
1. 分别创建三个循环链表,分别表示字母字符、数字字符和其他字符的线性表。
2. 从原线性链表的头节点开始,遍历整个链表。
3. 对于遍历到的每个节点,判断其所代表的字符属于哪一类。然后将该节点从原链表中删除,并将其添加到对应的循环链表的尾部。
4. 当原链表为空时,遍历结束。此时,三个循环链表中分别包含了字母字符、数字字符和其他字符的所有节点。
下面是一个简单的 Python 代码实现,供参考:
```
class Node:
def __init__(self, data):
self.data = data
self.next = None
def split_list(head):
letter_head = letter_tail = Node(None)
digit_head = digit_tail = Node(None)
other_head = other_tail = Node(None)
while head:
if head.data.isalpha():
letter_tail.next = head
letter_tail = letter_tail.next
elif head.data.isdigit():
digit_tail.next = head
digit_tail = digit_tail.next
else:
other_tail.next = head
other_tail = other_tail.next
head = head.next
letter_tail.next = letter_head.next
digit_tail.next = digit_head.next
other_tail.next = other_head.next
return letter_head.next, digit_head.next, other_head.next
```
上述代码中,我们通过创建四个节点来分别表示三个循环链表的尾节点以及它们的头节点。在遍历原链表时,将符合条件的节点添加到对应的循环链表尾部,同时将其从原链表中删除。最后,将每个循环链表的尾节点与头节点相连,形成循环链表。最后返回三个循环链表的头节点即可。
阅读全文
相关推荐


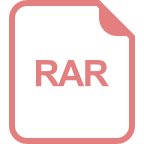
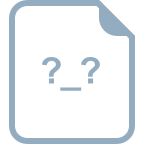
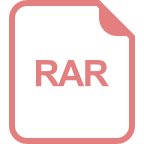
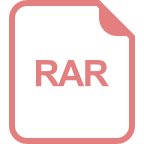
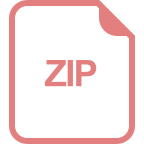
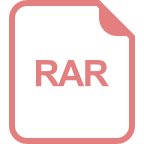
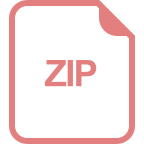
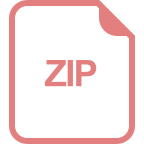
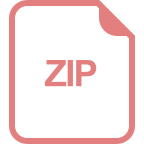
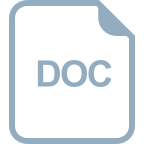
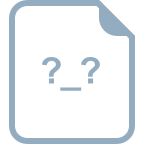