private void startGame() { Random random = new Random(); int n = random.nextInt(4) + 1; // 生成1-4之间的随机数 int totalCount = n * 25; // "处"的总数量 externalCount = totalCount / 25; // "外"的数量 StringBuilder sb = new StringBuilder(); for (int i = 0; i < totalCount; i++) { if (i < externalCount) { sb.append("外"); } else { sb.append("处"); } }这段代码知识点的讲解
时间: 2024-04-22 21:28:49 浏览: 97
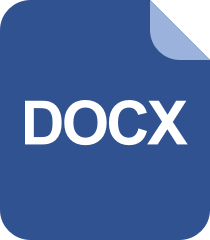
项目设计说明书1
这段代码是一个简单的Java方法startGame(),它使用了随机数生成器来生成一个1到4之间的随机整数n。然后,它使用n计算出了“处”字符和“外”字符的总数,并将“外”字符的数量存储在一个变量externalCount中。
接下来,使用一个StringBuilder对象sb来创建一个包含“处”和“外”字符的字符串。在循环中,如果当前的索引i小于externalCount,则将“外”字符添加到字符串中,否则将“处”字符添加到字符串中。
这段代码涉及的主要知识点包括:
1. 随机数生成器:Random类的nextInt()方法可以生成指定范围内的随机整数。
2. 字符串操作:StringBuilder类可以用于创建可变的字符串对象,它的append()方法可以用于向字符串中添加字符或字符串。
3. 循环:使用for循环可以重复执行一段代码块,直到满足退出条件为止。在这个例子中,循环从0到totalCount-1,每次循环使用if-else语句来决定向字符串中添加哪个字符。
阅读全文
相关推荐
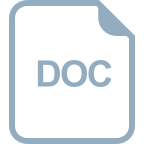
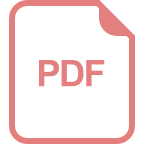













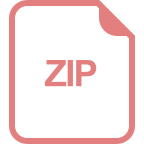
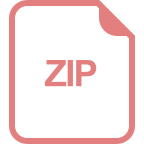