C++中哈希表如何遍历
时间: 2024-05-03 07:17:35 浏览: 423
在C++中,哈希表可以使用迭代器或范围循环进行遍历。
使用迭代器遍历哈希表的示例代码如下:
```c++
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> myMap = { {"apple", 1}, {"banana", 2}, {"orange", 3} };
// 使用迭代器遍历哈希表
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
```
使用范围循环遍历哈希表的示例代码如下:
```c++
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> myMap = { {"apple", 1}, {"banana", 2}, {"orange", 3} };
// 使用范围循环遍历哈希表
for (const auto& [key, value] : myMap) {
std::cout << key << ": " << value << std::endl;
}
return 0;
}
```
其中,使用auto关键字定义的迭代器it指向哈希表的第一个元素,每次遍历时先判断迭代器是否等于哈希表的end()迭代器,如果不等于,则输出当前元素的键值对,然后将迭代器指向下一个元素。使用范围循环则更加简洁,直接遍历哈希表的所有元素并输出。
阅读全文
相关推荐
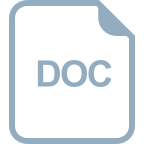
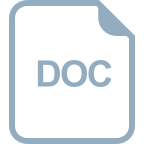
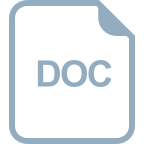
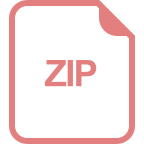
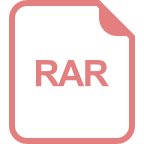













