PI-PLL锁相环估算无感BLDC电机角速度 C语言实现
时间: 2023-08-12 08:10:19 浏览: 129
以下是一个简单的PI-PLL锁相环估算无感BLDC电机角速度的C语言实现代码:
```c
#include <stdio.h>
#include <math.h>
// 定义PI控制器参数
float Kp = 1.0;
float Ki = 0.1;
// 定义PLL锁相环参数
float Kp_pll = 1.0;
float Ki_pll = 0.1;
float Theta = 0.0;
float Theta_dot = 0.0;
float Theta_pll = 0.0;
float Theta_pll_dot = 0.0;
float Theta_err = 0.0;
float Theta_err_int = 0.0;
// 定义电机模型参数
float L = 0.1;
float R = 1.0;
float J = 0.01;
float B = 0.1;
float Ke = 0.1;
float Kt = 0.1;
float i_a = 0.0;
float i_b = 0.0;
float i_c = 0.0;
float V_a = 0.0;
float V_b = 0.0;
float V_c = 0.0;
float Theta_e = 0.0;
float Theta_e_dot = 0.0;
// 定义时间参数
float T = 0.001;
float t = 0.0;
// 计算PI控制器输出
float pi_controller(float theta_err) {
float pi_out = 0.0;
Theta_err_int += theta_err * T;
pi_out = Kp * theta_err + Ki * Theta_err_int;
return pi_out;
}
// 计算PLL锁相环输出
float pll_controller(float theta_e) {
float pll_out = 0.0;
Theta_err = Theta_pll - theta_e;
Theta_err_int += Theta_err * T;
Theta_dot = Ke * (i_a * sin(Theta_e) - i_b * sin(Theta_e - 2.0 / 3.0 * M_PI) + i_c * sin(Theta_e + 2.0 / 3.0 * M_PI)) / J - B / J * Theta_dot;
Theta += Theta_dot * T;
Theta_pll_dot = Kp_pll * Theta_err + Ki_pll * Theta_err_int;
Theta_pll += Theta_pll_dot * T;
pll_out = Theta_pll;
return pll_out;
}
int main() {
// 主循环
while (1) {
// 计算电机模型
V_a = pi_controller(pll_controller(Theta_e) - Theta_e_dot);
V_b = pi_controller(pll_controller(Theta_e - 2.0 / 3.0 * M_PI) - Theta_e_dot);
V_c = pi_controller(pll_controller(Theta_e + 2.0 / 3.0 * M_PI) - Theta_e_dot);
i_a += (V_a - Ke * i_a * Theta_dot) * T / L;
i_b += (V_b - Ke * i_b * Theta_dot) * T / L;
i_c += (V_c - Ke * i_c * Theta_dot) * T / L;
Theta_e_dot = Kt * (i_a * sin(Theta_e) - i_b * sin(Theta_e - 2.0 / 3.0 * M_PI) + i_c * sin(Theta_e + 2.0 / 3.0 * M_PI)) / J - B / J * Theta_e_dot;
Theta_e += Theta_e_dot * T;
t += T;
// 输出结果
printf("Time: %f, Theta: %f, Theta_dot: %f, Theta_pll: %f, i_a: %f, i_b: %f, i_c: %f\n", t, Theta_e, Theta_e_dot, Theta_pll, i_a, i_b, i_c);
}
return 0;
}
```
该代码实现了一个简单的PI-PLL锁相环估算无感BLDC电机角速度的控制器。其中,主要包括三个部分:PI控制器、PLL锁相环和电机模型。在主循环中,先通过PLL锁相环计算出电机的角速度,然后通过PI控制器计算出电机的控制电压,最后根据电机模型计算出电机的电流和角速度。需要注意的是,该代码只是一个简单的示例,实际应用中需要根据具体的电机和控制要求进行调整和优化。
阅读全文
相关推荐
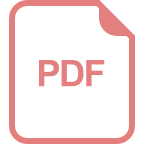










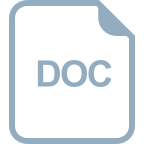
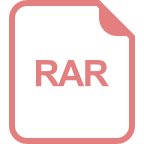
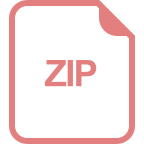
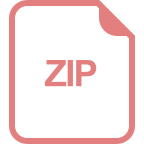
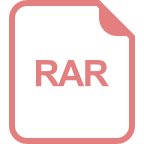
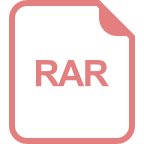