Python把路径中的多个csv文件合并成多个sheet,sheet名为相应表名的代码
时间: 2024-05-09 12:17:08 浏览: 12
可以使用pandas库中的concat函数,具体代码如下:
``` python
import pandas as pd
import glob
# 读取多个csv文件
all_files = glob.glob('path/to/files/*.csv')
# 通过循环读取并合并所有文件
sheets = {}
for filename in all_files:
df = pd.read_csv(filename)
sheet_name = filename.split('/')[-1].split('.')[0] # 获取表名
sheets[sheet_name] = df
# 将合并后的数据写入Excel文件
with pd.ExcelWriter('output_file.xlsx') as writer:
for sheet_name, sheet_data in sheets.items():
sheet_data.to_excel(writer, sheet_name=sheet_name, index=False)
```
这段代码会将指定路径下的所有csv文件读取并合并到一个字典对象中,以每个文件的文件名(不包含扩展名)作为sheet的名称,最后将所有sheet写入到一个Excel文件中。
相关问题
Python把路径中的excel格式是CVS的文件合并成多个sheet,sheet名为相应的表名的代码
可以使用 pandas 模块中的 read_csv() 和 ExcelWriter() 函数来实现。代码如下:
```
import pandas as pd
# 读取CSV文件
df = pd.read_csv('path/to/file.csv')
# 按照表名分组
groups = df.groupby('表名')
# 创建ExcelWriter对象
writer = pd.ExcelWriter('path/to/output/file.xlsx')
# 循环写入每个表的数据
for name, group in groups:
group.to_excel(writer, sheet_name=name, index=False)
# 保存Excel文件
writer.save()
```
这段代码会将 CSV 文件读取为 DataFrame 对象,然后按照表名分组,并创建一个 ExcelWriter 对象。最后循环写入每个表的数据到 Excel 文件中,每个表对应一个 sheet,sheet 名称为表名。
python合并多个csv为一个excel的多个sheet
可以使用 pandas 库来合并多个 CSV 文件,并将它们保存为一个 Excel 文件的多个 sheet。以下是一个示例代码:
```python
import pandas as pd
# 假设有三个 CSV 文件:file1.csv、file2.csv 和 file3.csv
file_names = ['file1.csv', 'file2.csv', 'file3.csv']
# 读取每个 CSV 文件,并将它们存储在一个列表中
dfs = [pd.read_csv(filename) for filename in file_names]
# 创建一个 Excel 写入器
writer = pd.ExcelWriter('output.xlsx')
# 将每个 DataFrame 存储为 Excel 的一个 sheet
for i, df in enumerate(dfs):
df.to_excel(writer, sheet_name=f'Sheet{i+1}', index=False)
# 保存 Excel 文件
writer.save()
```
这个代码会将三个 CSV 文件合并到一个名为 `output.xlsx` 的 Excel 文件中,并将它们分别存储在名为 `Sheet1`、`Sheet2` 和 `Sheet3` 的 sheet 中。你可以根据需要修改代码,以适应你的具体情况。
相关推荐
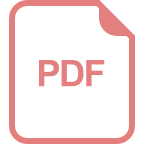
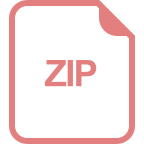












