cv2.houghlines
时间: 2023-09-04 16:16:10 浏览: 43
cv2.HoughLines() 是一个在图像中检测直线的函数,它基于霍夫变换(Hough Transform)。该函数需要一个二值化图像作为输入,然后输出一组检测到的直线参数(rho 和 theta),以及可选的直线的端点。
函数的语法如下:
```
lines = cv2.HoughLines(image, rho, theta, threshold[, lines[, srn[, stn[, min_theta[, max_theta]]]]])
```
参数说明:
- image:输入的二值化图像
- rho:距离参数 rho 的精度,以像素为单位
- theta:角度参数 theta 的精度,以弧度为单位
- threshold:阈值参数,只有当累加器中的值高于 threshold 时,才被认为是一条直线
- lines:可选的输出参数,检测到的直线参数 (rho, theta) 的 numpy 数组
- srn 和 stn:可选的参数,用于减少计算量,具体含义请参考 OpenCV 文档
- min_theta 和 max_theta:可选的参数,表示直线角度的最小值和最大值,超出这个范围的直线将被忽略。
相关问题
cv2.HoughLines
`cv2.HoughLines`是OpenCV中实现霍夫变换的函数之一,用于检测图像中的直线。该函数的用法如下:
```python
lines = cv2.HoughLines(image, rho, theta, threshold)
```
其中,参数说明如下:
- `image`: 输入图像,必须为单通道二值图像。
- `rho`: 霍夫空间中的距离分辨率,一般设为1。
- `theta`: 霍夫空间中的角度分辨率,一般设为np.pi/180。
- `threshold`: 霍夫变换的阈值,即检测到的直线必须满足的最小投票数。该值越大,检测到的直线越明显,但可能会漏掉一些直线。
该函数返回一个`lines`数组,其中每个元素为一个包含两个值的数组,分别表示直线在极坐标空间中的距离和角度。
值得注意的是,`cv2.HoughLines`函数只能检测到直线,如果要检测其他形状的曲线,需要使用`cv2.HoughLinesP`函数。
cv2.houghlines和cv2.HoughLinesP、
`cv2.HoughLines` 和 `cv2.HoughLinesP` 都是 OpenCV 中用于直线检测的函数,但它们在参数和功能上有所不同。
`cv2.HoughLines` 函数使用标准的霍夫变换算法来检测直线。它返回的是检测到的直线的参数 rho 和 theta。需要注意的是,`cv2.HoughLines` 函数只能检测出直线的参数,需要进一步处理才能绘制出直线或者获取直线的端点坐标。
`cv2.HoughLinesP` 函数使用改进的霍夫变换算法来检测直线,称为随机霍夫变换。相比于 `cv2.HoughLines`,`cv2.HoughLinesP` 返回的是直线的端点坐标,而不仅仅是直线的参数 rho 和 theta。这样可以更方便地绘制直线或者进行后续处理。
函数调用的语法如下:
```python
lines = cv2.HoughLinesP(image, rho, theta, threshold, minLineLength, maxLineGap)
```
- `image`:输入的二值化图像,通常是通过 Canny 边缘检测等方法得到的。
- `rho`:以像素为单位的距离精度。一般设为 1。
- `theta`:以弧度为单位的角度精度。一般设为 np.pi/180。
- `threshold`:阈值参数,用于确定检测到直线的最小投票数。
- `minLineLength`:最小直线长度,比这个长度短的直线将被丢弃。
- `maxLineGap`:最大直线间隙,如果两条直线之间的间隙大于这个值,则被认为是两条不同的直线。
需要注意的是,`cv2.HoughLinesP` 函数返回的直线是以端点坐标 (x1, y1, x2, y2) 表示的。
相关推荐
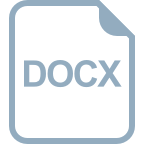












