判断是否为avl树
时间: 2023-08-28 11:06:40 浏览: 143
判断一棵二叉树是否为 AVL 树,需要满足以下两个条件:
1. 该二叉树的所有子树都是 AVL 树;
2. 该二叉树的任意两个子树高度差的绝对值不超过 1。
其中,子树的高度指的是该子树的根节点到叶子节点的最长路径长度。
因此,判断一棵二叉树是否为 AVL 树,需要对该二叉树进行递归遍历,依次判断每个子树是否为 AVL 树,并计算每个子树的高度,最后再判断任意两个子树高度差的绝对值是否不超过 1。如果满足以上两个条件,则该二叉树为 AVL 树,否则不是 AVL 树。
具体实现过程可以参考以下代码(假设二叉树的节点结构为 Node,其包含 left、right、height 三个属性):
```python
def is_avl_tree(root):
# 辅助函数,计算节点高度
def get_height(node):
if not node:
return 0
return node.height
# 判断一棵二叉树是否为 AVL 树
def is_avl_tree_helper(node):
if not node:
return True
# 判断左右子树是否为 AVL 树
if not is_avl_tree_helper(node.left) or not is_avl_tree_helper(node.right):
return False
# 计算左右子树的高度差
height_diff = abs(get_height(node.left) - get_height(node.right))
if height_diff > 1:
return False
# 更新当前节点的高度
node.height = max(get_height(node.left), get_height(node.right)) + 1
return True
# 判断根节点是否为空,为空则返回 False
if not root:
return False
return is_avl_tree_helper(root)
```
注意,以上代码仅为示例,具体实现可能因语言和实际情况的不同而有所差异。
阅读全文
相关推荐
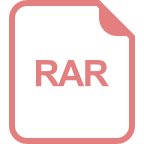
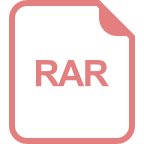
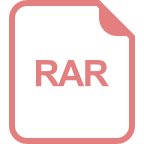
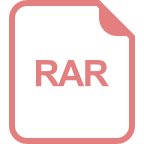

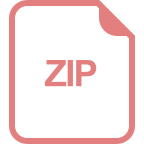
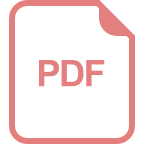
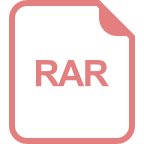
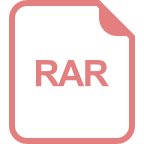
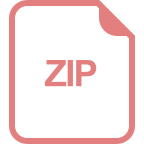
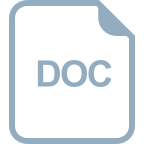
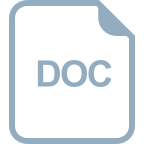
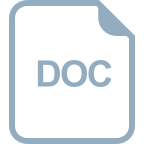
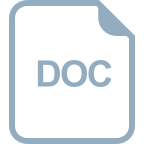
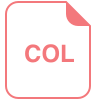
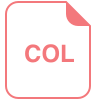