分支限界01背包问题c语言
时间: 2023-08-12 09:22:01 浏览: 44
好的,那我来给你讲一下分支限界法解决01背包问题的思路。
首先,我们需要定义一个节点结构体,来记录每一次放置物品的状态。具体代码如下:
```c
typedef struct node {
int level; // 当前节点的深度
int profit; // 当前节点已经获得的价值
int weight; // 当前节点已经放置的物品重量
float bound; // 当前节点的上界
} node;
```
然后,我们需要定义一个比较函数,用来对节点进行排序。这里我选择按照节点的上界进行排序,代码如下:
```c
int cmp(const void *a, const void *b) {
node *p = (node *)a;
node *q = (node *)b;
if (p->bound < q->bound) {
return 1;
} else if (p->bound > q->bound) {
return -1;
} else {
return 0;
}
}
```
接着,我们需要编写一个函数,来计算当前节点的上界。这里我选择使用贪心算法来计算上界,代码如下:
```c
float bound(node u, int n, int *w, int *p, int c) {
if (u.weight >= c) {
return 0;
}
float bound = u.profit;
int j = u.level + 1;
int totweight = u.weight;
while (j <= n && totweight + w[j] <= c) {
totweight += w[j];
bound += p[j];
j++;
}
if (j <= n) {
bound += (c - totweight) * (p[j] * 1.0 / w[j]);
}
return bound;
}
```
最后,我们只需要编写一个函数,来实现分支限界法即可。代码如下:
```c
int knapsack(int n, int *w, int *p, int c) {
int maxprofit = 0;
node u, v;
node q[n + 1];
q[0].level = 0;
q[0].profit = 0;
q[0].weight = 0;
q[0].bound = bound(q[0], n, w, p, c);
int head = 0, tail = 0;
qsort(q, n + 1, sizeof(node), cmp);
tail++;
while (head < tail) {
u = q[head];
if (u.bound > maxprofit) {
v.level = u.level + 1;
v.weight = u.weight + w[v.level];
v.profit = u.profit + p[v.level];
v.bound = bound(v, n, w, p, c);
if (v.weight <= c && v.profit > maxprofit) {
maxprofit = v.profit;
}
if (v.bound > maxprofit) {
q[tail] = v;
tail++;
qsort(q + head + 1, tail - head - 1, sizeof(node), cmp);
}
v.weight = u.weight;
v.profit = u.profit;
v.bound = bound(v, n, w, p, c);
if (v.bound > maxprofit) {
q[tail] = v;
tail++;
qsort(q + head + 1, tail - head - 1, sizeof(node), cmp);
}
}
head++;
}
return maxprofit;
}
```
这个函数的返回值就是01背包问题的最大价值了。
希望这段代码对你有所帮助!
相关推荐
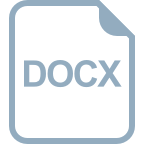














