// 创建开始按钮 startButton = new JButton("开始游戏"); startButton.addActionListener(this); gamePanel.add(startButton, BorderLayout.CENTER); // 将游戏面板添加到窗口中 getContentPane().add(gamePanel); // 设置窗口大小和关闭操作 setSize(400, 300); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public void actionPerformed(ActionEvent e) { // 点击开始按钮后开始游戏 if (e.getSource() == startButton) { seletMenu(); } } public void seletMenu() { JPanel panel= new JPanel(); SimpleButton = new JButton("娱乐模式"); SimpleButton.addActionListener(this); panel.add(SimpleButton); HardButton = new JButton("挑战模式"); HardButton.addActionListener(this); panel.add(HardButton); setVisible(true); }
时间: 2024-03-18 15:39:40 浏览: 83
根据您提供的代码,可能是因为在 `seletMenu()` 方法中,没有将 `panel` 添加到窗口中。您需要将 `panel` 添加到窗口中才能显示菜单。可以在 `seletMenu()` 方法的最后添加以下代码:
```
add(panel);
revalidate();
repaint();
```
其中,`add(panel)` 将 `panel` 添加到窗口中,`revalidate()` 方法重新验证窗口的布局,`repaint()` 方法重新绘制窗口。这些操作应该足以显示您的菜单。
相关问题
ChengYu currentChengYu = null; System.out.println("进入娱乐模式"); chengYuList = commonChengYuList; Collections.shuffle(chengYuList); // 打乱顺序 for (ChengYu cy : chengYuList) { if (currentChengYu == null) { for (ChengYu cy4 : fullChengYuList) { if (cy4.getChengYu() .startsWith((cy.getChengYu().substring(cy.getChengYu().length() - 1)))) { currentChengYu = cy; break; } } } if (currentChengYu != null) { cy = currentChengYu; } currentIdiom = cy.getChengYu(); System.out.println("请回答以下成语的下一个成语:" + currentIdiom); String answer = scanner.next(); if (answer == null) break; if (!cy.isCorrectAnswerEasy(answer, fullChengYuList)) { System.out.println("回答错误!"); success = false; getHint(cy.getChengYu()); currentChengYu = null; if (hintCount >= maxHintCount) { System.out.println("提示次数已全部用完,请重新开始游戏!"); hintCount = 0; break; } else continue; } else { score++; System.out.println("答对加1分"); success = true; } if (success) { boolean label = true; System.out.println("现在的得分为:" + score); for (ChengYu nextChengYu : chengYuList) { if (nextChengYu.getChengYu().startsWith((answer.substring(answer.length() - 1)))) { for (ChengYu cy2 : chengYuList) { if (cy2.getChengYu() .startsWith((nextChengYu.getChengYu() .substring(nextChengYu.getChengYu().length() - 1))) && nextChengYu != currentChengYu) { currentChengYu = nextChengYu; label = false; break; } } ; } } if (label) { currentChengYu = null; System.out.println("无法继续接龙"); continue; } } } if (score == 16) System.out.print("恭喜通关,你真是个小聪明");在这个代码中添加一些java swing库的应用,要求能够实现窗口
可以使用Java Swing库创建一个简单的窗口,并将游戏界面放入其中。以下是一个简单的示例代码:
```
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JLabel;
import javax.swing.JButton;
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ChengYuGame extends JFrame implements ActionListener {
private JPanel gamePanel;
private JLabel scoreLabel;
private JButton startButton;
private int score;
public ChengYuGame() {
// 设置窗口标题
super("成语接龙游戏");
// 创建游戏面板
gamePanel = new JPanel();
gamePanel.setLayout(new BorderLayout());
// 创建得分标签
scoreLabel = new JLabel("得分: " + score);
gamePanel.add(scoreLabel, BorderLayout.NORTH);
// 创建开始按钮
startButton = new JButton("开始游戏");
startButton.addActionListener(this);
gamePanel.add(startButton, BorderLayout.CENTER);
// 将游戏面板添加到窗口中
getContentPane().add(gamePanel);
// 设置窗口大小和关闭操作
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void actionPerformed(ActionEvent e) {
// 点击开始按钮后开始游戏
if (e.getSource() == startButton) {
playGame();
}
}
private void playGame() {
// TODO: 添加游戏逻辑
}
public static void main(String[] args) {
// 创建游戏窗口
ChengYuGame game = new ChengYuGame();
game.setVisible(true);
}
}
```
在这个示例代码中,我们创建了一个`JFrame`窗口,并在其中添加了一个`JPanel`面板作为游戏界面。面板中包含一个得分标签和一个开始按钮。当用户点击开始按钮时,将调用`playGame()`方法开始游戏。我们可以在`playGame()`方法中添加原先的游戏逻辑。在游戏中,我们可以通过更新得分标签来显示当前得分,例如:`scoreLabel.setText("得分: " + score)`。
用java编写井字游戏“Tictactoe.java”,该页面带有简单的菜单栏,且只有一个“操作”菜单,下含“开始游戏”,“保存游戏”,“退出游戏”三个菜单条,游戏界面包括下拉框——九宫格选项(33,44;...10*10),页面可随用户选择变换大小,有“开始”,“再来一局”按钮:要求程序启动后,程序界面为灰色,需要启动菜单栏的开始游戏才能允许九宫格选项,点击开始/再来一局按钮游戏开始对战,双方各执“X”,"O"不同符号表示对战
好的,下面是一个基本的实现示例:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Tictactoe extends JFrame {
private static final long serialVersionUID = 1L;
private JPanel gamePanel;
private JComboBox<String> sizeComboBox;
private JButton startButton;
private JButton restartButton;
private JMenuItem startMenuItem;
private JMenuItem saveMenuItem;
private JMenuItem exitMenuItem;
private JFileChooser fileChooser;
private char[][] board;
private char currentPlayer;
private boolean gameEnded;
public Tictactoe() {
// 设置窗口标题和大小
super("Tictactoe");
setSize(400, 400);
// 创建菜单栏和菜单条
JMenuBar menuBar = new JMenuBar();
JMenu operationMenu = new JMenu("操作");
menuBar.add(operationMenu);
setJMenuBar(menuBar);
// 创建菜单项并添加到菜单条中
startMenuItem = new JMenuItem("开始游戏");
operationMenu.add(startMenuItem);
saveMenuItem = new JMenuItem("保存游戏");
operationMenu.add(saveMenuItem);
exitMenuItem = new JMenuItem("退出游戏");
operationMenu.add(exitMenuItem);
// 为菜单项添加事件处理程序
startMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
startGame();
}
});
saveMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
saveGame();
}
});
exitMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.exit(0);
}
});
// 创建下拉框并添加到内容面板中
String[] sizes = {"3x3", "4x4", "5x5", "6x6", "7x7", "8x8", "9x9", "10x10"};
sizeComboBox = new JComboBox<>(sizes);
sizeComboBox.setEnabled(false);
JPanel controlPanel = new JPanel(new FlowLayout());
controlPanel.add(new JLabel("九宫格大小:"));
controlPanel.add(sizeComboBox);
startButton = new JButton("开始");
startButton.setEnabled(false);
startButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
startButton.setEnabled(false);
sizeComboBox.setEnabled(false);
gamePanel.removeAll();
gamePanel.setLayout(new GridLayout(getBoardSize(), getBoardSize()));
board = new char[getBoardSize()][getBoardSize()];
currentPlayer = 'X';
gameEnded = false;
for (int i = 0; i < getBoardSize(); i++) {
for (int j = 0; j < getBoardSize(); j++) {
JButton button = new JButton();
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if (!gameEnded) {
JButton clickedButton = (JButton) e.getSource();
int row = getButtonRow(clickedButton);
int col = getButtonCol(clickedButton);
if (board[row][col] == '\0') {
board[row][col] = currentPlayer;
clickedButton.setText("" + currentPlayer);
if (checkWin(row, col)) {
JOptionPane.showMessageDialog(Tictactoe.this, "恭喜" + currentPlayer + "获胜!");
gameEnded = true;
startButton.setEnabled(true);
} else if (checkTie()) {
JOptionPane.showMessageDialog(Tictactoe.this, "平局!");
gameEnded = true;
startButton.setEnabled(true);
} else {
currentPlayer = (currentPlayer == 'X') ? 'O' : 'X';
}
}
}
}
});
gamePanel.add(button);
}
}
gamePanel.revalidate();
gamePanel.repaint();
}
});
controlPanel.add(startButton);
restartButton = new JButton("再来一局");
restartButton.setEnabled(false);
restartButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
for (int i = 0; i < getBoardSize(); i++) {
for (int j = 0; j < getBoardSize(); j++) {
board[i][j] = '\0';
}
}
for (Component c : gamePanel.getComponents()) {
JButton button = (JButton) c;
button.setText("");
}
currentPlayer = 'X';
gameEnded = false;
restartButton.setEnabled(false);
}
});
controlPanel.add(restartButton);
add(controlPanel, BorderLayout.NORTH);
// 创建游戏面板并添加到内容面板中
gamePanel = new JPanel();
gamePanel.setBackground(Color.GRAY);
add(gamePanel, BorderLayout.CENTER);
// 创建文件选择器
fileChooser = new JFileChooser();
}
private int getBoardSize() {
String size = (String) sizeComboBox.getSelectedItem();
return Integer.parseInt(size.substring(0, size.indexOf('x')));
}
private int getButtonRow(JButton button) {
return gamePanel.getComponentZOrder(button) / getBoardSize();
}
private int getButtonCol(JButton button) {
return gamePanel.getComponentZOrder(button) % getBoardSize();
}
private boolean checkWin(int row, int col) {
// 检查行
for (int i = 0; i < getBoardSize(); i++) {
if (board[row][i] != currentPlayer) {
break;
}
if (i == getBoardSize() - 1) {
return true;
}
}
// 检查列
for (int i = 0; i < getBoardSize(); i++) {
if (board[i][col] != currentPlayer) {
break;
}
if (i == getBoardSize() - 1) {
return true;
}
}
// 检查对角线
if (row == col) {
for (int i = 0; i < getBoardSize(); i++) {
if (board[i][i] != currentPlayer) {
break;
}
if (i == getBoardSize() - 1) {
return true;
}
}
}
if (row + col == getBoardSize() - 1) {
for (int i = 0; i < getBoardSize(); i++) {
if (board[i][getBoardSize() - i - 1] != currentPlayer) {
break;
}
if (i == getBoardSize() - 1) {
return true;
}
}
}
return false;
}
private boolean checkTie() {
for (int i = 0; i < getBoardSize(); i++) {
for (int j = 0; j < getBoardSize(); j++) {
if (board[i][j] == '\0') {
return false;
}
}
}
return true;
}
private void startGame() {
sizeComboBox.setEnabled(true);
}
private void saveGame() {
int result = fileChooser.showSaveDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
// TODO: 保存游戏状态到文件
}
}
public static void main(String[] args) {
Tictactoe game = new Tictactoe();
game.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
game.setVisible(true);
}
}
```
在这个示例中,我们创建了一个Tictactoe类作为游戏的主类,继承自JFrame类。在构造函数中,我们创建了菜单栏、下拉框、按钮和游戏面板,并且为菜单项和按钮添加了事件处理程序。我们使用二维数组来表示游戏面板,并且使用GridLayout布局管理器来动态改变游戏面板的大小。当用户点击一个按钮时,我们检查游戏是否结束,如果没有结束,我们更新游戏状态并检查是否有获胜者或平局。最后,我们使用文件选择器来实现保存游戏状态的功能。
请注意,这只是一个基本的实现示例,您可能需要根据您的具体需求进行修改和扩展。
阅读全文
相关推荐
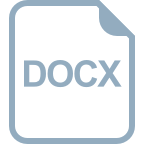
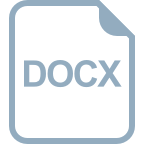










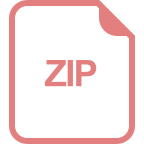
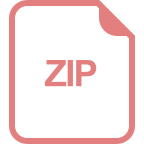