in_data = input.transpose([0, 3, 1, 2]).to(device) AttributeError: 'Tensor' object has no attribute 'to'
时间: 2024-05-11 13:18:11 浏览: 13
这个错误通常是因为你的 Tensor 对象不是 PyTorch 的 Tensor 对象,而是其他库的 Tensor 对象,比如 NumPy 的 ndarray。PyTorch 的 Tensor 对象有 to() 方法,但其他库的 Tensor 对象没有这个方法。
要解决这个问题,你需要将你的 Tensor 对象转换为 PyTorch 的 Tensor 对象。你可以使用 torch.from_numpy() 方法来将 NumPy 的 ndarray 对象转换为 PyTorch 的 Tensor 对象:
```python
import torch
import numpy as np
# 假设 in_data 是一个 NumPy 的 ndarray 对象
in_data = np.array([[1,2,3], [4,5,6]])
tensor_data = torch.from_numpy(in_data)
tensor_data = tensor_data.transpose(0, 1) # 转换维度
tensor_data = tensor_data.to(device) # 将 Tensor 对象移到 GPU 上(如果有的话)
```
如果你的 Tensor 对象不是 NumPy 的 ndarray 对象,而是其他库的 Tensor 对象,你需要查看该库提供的转换方法。
相关问题
paddle 2.2.2提示 in_data = input.permute(0, 3, 1, 2).to(device) AttributeError: 'Tensor' object has no attribute 'permute'
这个错误提示说 Tensor 对象没有 `permute` 属性,通常是因为你使用的版本太旧了,或者你使用的不是 Tensor 对象。在 PaddlePaddle 2.x 版本中,`permute` 方法已经被重命名为 `transpose`。你可以将 `permute` 方法替换为 `transpose` 方法,例如将
```python
in_data = input.permute(0, 3, 1, 2).to(device)
```
替换为
```python
in_data = input.transpose([0, 3, 1, 2]).to(device)
```
注意,`transpose` 方法中传入的参数是一个列表,而不是多个参数。如果你使用的不是 Tensor 对象,那么请检查一下输入的对象类型是否正确。
这是什么意思 input0_data = np.transpose(input0_data, (1, 2, 0, 3))
这段代码使用了`np.transpose`函数对`input0_data`进行转置操作。转置操作可以改变数组的维度顺序。
具体来说,`(1, 2, 0, 3)`是一个元组,表示新的维度顺序。在原数组`input0_data`中,它的维度顺序可能是`(0, 1, 2, 3)`,而通过转置操作,将第0维移到第2个位置,第1维移到第0个位置,第2维移到第1个位置,第3维保持不变,得到了新的数组。
这种操作常用于调整数据的维度顺序以适应不同的计算需求或数据格式要求。在这段代码中,可能是为了满足某个模型或库对输入数据维度顺序的要求。
相关推荐
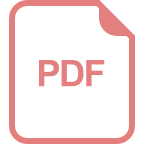
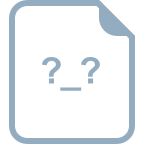












