android 使用Java语言展示3D模型具体实现代码 以及使用到的依赖
时间: 2024-03-05 18:52:27 浏览: 22
展示3D模型的Android应用可以使用OpenGL ES或Unity等技术实现。以下是使用OpenGL ES展示3D模型的示例代码和依赖。
依赖:
```gradle
implementation 'com.android.support:appcompat-v7:28.0.0'
implementation 'com.android.support.constraint:constraint-layout:1.1.3'
implementation 'com.android.support:support-v4:28.0.0'
implementation 'com.android.support:design:28.0.0'
implementation 'javax.inject:javax.inject:1'
implementation 'org.greenrobot:eventbus:3.1.1'
implementation 'com.google.code.gson:gson:2.8.5'
implementation 'com.squareup.retrofit2:retrofit:2.6.2'
implementation 'com.squareup.retrofit2:converter-gson:2.6.2'
implementation 'com.squareup.okhttp3:okhttp:3.12.1'
implementation 'com.squareup.okhttp3:logging-interceptor:3.12.1'
implementation 'io.reactivex.rxjava2:rxandroid:2.1.1'
implementation 'io.reactivex.rxjava2:rxjava:2.2.10'
implementation 'com.jakewharton.rxbinding2:rxbinding:2.1.1'
implementation 'com.jakewharton:butterknife:10.1.0'
kapt 'com.jakewharton:butterknife-compiler:10.1.0'
```
Java代码:
```java
public class GLView extends GLSurfaceView implements Renderer {
private Context mContext;
private ObjLoader objLoader;
private int texture;
private float mScale = 1f;
private float mXAngle = 0f;
private float mYAngle = 0f;
private float mZAngle = 0f;
public GLView(Context context) {
super(context);
mContext = context;
setEGLContextClientVersion(2);
setRenderer(this);
setRenderMode(GLSurfaceView.RENDERMODE_WHEN_DIRTY);
}
public GLView(Context context, AttributeSet attrs) {
super(context, attrs);
mContext = context;
setEGLContextClientVersion(2);
setRenderer(this);
setRenderMode(GLSurfaceView.RENDERMODE_WHEN_DIRTY);
}
@Override
public void onSurfaceCreated(GL10 gl, EGLConfig config) {
GLES20.glClearColor(0.0f, 0.0f, 0.0f, 0.0f);
GLES20.glEnable(GLES20.GL_DEPTH_TEST);
GLES20.glEnable(GLES20.GL_TEXTURE_2D);
objLoader = new ObjLoader(mContext, "3d_model.obj");
texture = TextureUtils.initTexture(mContext, "texture.png");
}
@Override
public void onSurfaceChanged(GL10 gl, int width, int height) {
GLES20.glViewport(0, 0, width, height);
float ratio = (float) width / height;
Matrix.frustumM(mProjectMatrix, 0, -ratio, ratio, -1, 1, 1, 20);
Matrix.setLookAtM(mViewMatrix, 0, 0, 0, 3, 0, 0, 0, 0, 1, 0);
}
@Override
public void onDrawFrame(GL10 gl) {
GLES20.glClear(GLES20.GL_COLOR_BUFFER_BIT | GLES20.GL_DEPTH_BUFFER_BIT);
Matrix.setIdentityM(mModelMatrix, 0);
Matrix.scaleM(mModelMatrix, 0, mScale, mScale, mScale);
Matrix.rotateM(mModelMatrix, 0, mXAngle, 1, 0, 0);
Matrix.rotateM(mModelMatrix, 0, mYAngle, 0, 1, 0);
Matrix.rotateM(mModelMatrix, 0, mZAngle, 0, 0, 1);
objLoader.draw(texture, mModelMatrix, mViewMatrix, mProjectMatrix);
}
}
```
其中,`ObjLoader`是自定义的3D模型加载器类,`TextureUtils`是自定义的纹理加载器类。可以根据实际情况进行修改。
相关推荐
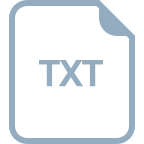
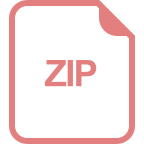
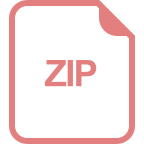














