删除容器内std::vector<Point3d>& Vec指定的点
时间: 2024-03-22 15:38:43 浏览: 30
好的,针对您的问题,我可以提供一个简单的实现思路:
1. 遍历 std::vector<Point3d>& Vec,找到所有需要删除的点的下标。
2. 根据找到的下标,将需要删除的点从 std::vector<Point3d>& Vec 中移除。
3. 返回删除后的 std::vector<Point3d>& Vec。
以下是一个样例代码实现,仅供参考:
```
void RemovePoints(std::vector<Point3d>& Vec, std::vector<int> IndicesToRemove)
{
// 根据下标从小到大排序
std::sort(IndicesToRemove.begin(), IndicesToRemove.end());
// 从后往前删除需要删除的点
for (int i = IndicesToRemove.size() - 1; i >= 0; i--)
{
Vec.erase(Vec.begin() + IndicesToRemove[i]);
}
}
```
注意,这只是一个简单的实现,您可以根据自己的实际需求进行修改。同时,为了保证代码的正确性和安全性,建议您在实际使用中进行充分的测试。
相关问题
移除容器内vector<Point3d>&Vec指定公差的点 ,代码如何写
好的,根据你的需求,我可以提供以下代码示例来移除vector<Point3d>&Vec中符合指定公差要求的点:
```c++
#include <vector>
#include <cmath>
struct Point3d {
double x, y, z;
};
void removePointsWithTolerance(std::vector<Point3d>& Vec, double tolerance) {
if (Vec.size() < 2) {
return;
}
std::vector<Point3d>::iterator it = Vec.begin();
Point3d lastPoint = *it;
++it;
while (it != Vec.end()) {
Point3d currentPoint = *it;
double distance = std::sqrt(
std::pow(currentPoint.x - lastPoint.x, 2) +
std::pow(currentPoint.y - lastPoint.y, 2) +
std::pow(currentPoint.z - lastPoint.z, 2));
if (distance < tolerance) {
it = Vec.erase(it);
}
else {
lastPoint = currentPoint;
++it;
}
}
}
```
这段代码会遍历vector<Point3d>&Vec中的所有点,并且计算出相邻两点之间的距离。如果距离小于指定公差tolerance,则会删除该点。如果距离大于等于指定公差tolerance,则会将该点作为下一个点的起点继续遍历。最后,vector<Point3d>&Vec中符合指定公差要求的点都被移除了。
你可以根据自己的需求修改代码中的数据类型和参数,并且根据需要进行错误处理和边界判断。
移除容器内vector<Point3d>&Vec指定公差的点
好的,针对您的问题,可以按照以下步骤来移除容器内指定公差的点:
1. 对容器内的点按照某种规则排序,例如按照点的 x 坐标排序。
2. 遍历容器内的点,对于相邻的两个点,如果它们之间的距离小于指定公差,就删除后面的点。
3. 遍历完成后,容器内就只剩下满足指定公差的点了。
具体实现代码如下:
```cpp
#include <vector>
#include <opencv2/core.hpp>
void removePointsWithTolerance(std::vector<cv::Point3d>& Vec, const double tolerance)
{
// 按照 x 坐标排序
std::sort(Vec.begin(), Vec.end(), [](const cv::Point3d& p1, const cv::Point3d& p2) {
return p1.x < p2.x;
});
// 遍历容器内的点,删除距离小于指定公差的点
auto it = Vec.begin();
while (it != Vec.end() - 1) {
const double dist = cv::norm(*it - *(it + 1)); // 计算两点之间的距离
if (dist < tolerance) {
it = Vec.erase(it + 1); // 删除后面的点
} else {
++it;
}
}
}
```
上述代码中,我们使用了 OpenCV 的 norm 函数来计算两点之间的距离,它可以计算任意维度的向量之间的距离。在实际使用中,需要根据具体情况来选择排序规则和距离计算方法。
相关推荐
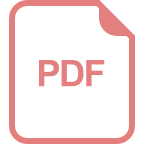
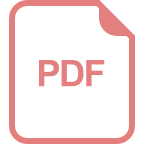
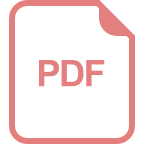












