C++实现贝塞尔曲线代码
时间: 2023-10-20 20:07:37 浏览: 51
以下是一个简单的 C 代码实现贝塞尔曲线:
```c
#include <stdio.h>
#include <math.h>
#define MAX_POINTS 100
typedef struct {
double x;
double y;
} Point;
Point points[MAX_POINTS];
int num_points = 0;
Point bezier(Point *p, double t) {
Point q;
double mt = 1 - t;
q.x = mt * mt * p[0].x + 2 * mt * t * p[1].x + t * t * p[2].x;
q.y = mt * mt * p[0].y + 2 * mt * t * p[1].y + t * t * p[2].y;
return q;
}
void plot_curve(Point *p) {
double t;
Point q;
for (t = 0; t <= 1; t += 0.01) {
q = bezier(p, t);
printf("%lf %lf\n", q.x, q.y);
}
}
int main() {
Point p[3] = {{50, 50}, {150, 200}, {250, 50}};
plot_curve(p);
return 0;
}
```
该代码定义了一个 `Point` 结构体,用于存储二维平面上的坐标。同时,还定义了一个 `bezier()` 函数,该函数用于计算贝塞尔曲线上某一点的坐标。最后,`main()` 函数中定义了三个控制点,并调用 `plot_curve()` 函数绘制贝塞尔曲线。
需要注意的是,该代码仅绘制了三个控制点的贝塞尔曲线。如果需要绘制更复杂的曲线,需要传入更多的控制点,并相应地修改 `bezier()` 函数的实现。
相关推荐
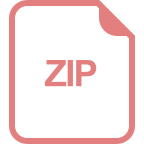
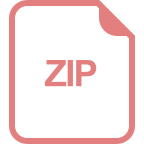














