c语言实现已知数据六个字母,把这些字母和频率作为叶子结点及权值计算带权路径长度WPL
时间: 2024-02-25 12:59:10 浏览: 25
可以使用哈夫曼树来实现这个问题。具体步骤如下:
1. 将六个字母按照出现频率从小到大排序。
2. 将频率最小的两个字母作为左右子节点,权值为两个字母的频率之和,构造一个新的二叉树。
3. 将新构造的二叉树作为一棵子树,继续从剩余的节点中选出频率最小的两个字母,构造新的二叉树并加入原二叉树中。
4. 重复步骤3,直到所有节点都被加入二叉树中,构造出哈夫曼树。
5. 计算带权路径长度WPL,即将每个叶子节点的权值乘以其到根节点的路径长度,再将所有叶子节点的计算结果相加。
以下是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct huffman_tree_node {
char data; // 字母
int weight; // 频率
struct huffman_tree_node *left_child, *right_child; // 左右子节点
} huffman_tree_node;
// 求哈夫曼树的带权路径长度
int calc_wpl(huffman_tree_node *root, int depth) {
if (root->left_child == NULL && root->right_child == NULL) {
return depth * root->weight;
}
return calc_wpl(root->left_child, depth + 1) + calc_wpl(root->right_child, depth + 1);
}
int main() {
char letters[] = {'A', 'B', 'C', 'D', 'E', 'F'};
int weights[] = {4, 6, 8, 12, 14, 16}; // 对应字母的频率
int n = sizeof(letters) / sizeof(letters[0]);
// 构造哈夫曼树
huffman_tree_node *nodes[n];
for (int i = 0; i < n; i++) {
nodes[i] = (huffman_tree_node *) malloc(sizeof(huffman_tree_node));
nodes[i]->data = letters[i];
nodes[i]->weight = weights[i];
nodes[i]->left_child = NULL;
nodes[i]->right_child = NULL;
}
while (n > 1) {
// 找到两个权值最小的节点
int min1 = 0, min2 = 1;
if (nodes[min1]->weight > nodes[min2]->weight) {
min1 = 1;
min2 = 0;
}
for (int i = 2; i < n; i++) {
if (nodes[i]->weight < nodes[min1]->weight) {
min2 = min1;
min1 = i;
} else if (nodes[i]->weight < nodes[min2]->weight) {
min2 = i;
}
}
// 构造新节点
huffman_tree_node *new_node = (huffman_tree_node *) malloc(sizeof(huffman_tree_node));
new_node->weight = nodes[min1]->weight + nodes[min2]->weight;
new_node->left_child = nodes[min1];
new_node->right_child = nodes[min2];
// 将新节点加入节点数组
nodes[min1] = new_node;
for (int i = min2; i < n - 1; i++) {
nodes[i] = nodes[i + 1];
}
n--;
}
// 计算哈夫曼树的带权路径长度
printf("WPL: %d\n", calc_wpl(nodes[0], 0));
return 0;
}
```
相关推荐
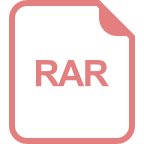
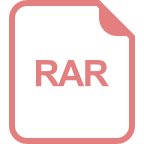
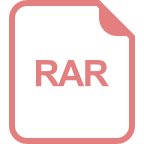












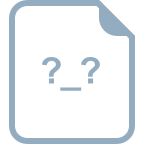
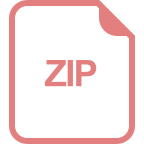
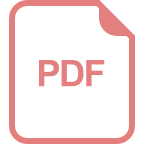