用processing代码做个小游戏
时间: 2023-09-04 13:07:57 浏览: 98
好的,我可以帮你写一个简单的井字棋游戏,使用Processing编写。以下是代码:
```java
// 定义格子大小
int CELL_SIZE = 100;
// 定义棋盘
char[][] board = new char[3][3];
char currentPlayer = 'X';
void setup() {
size(300, 300);
stroke(0);
strokeWeight(4);
textAlign(CENTER, CENTER);
textSize(50);
initBoard();
}
void draw() {
background(255);
drawBoard();
drawPieces();
checkWin();
}
// 初始化棋盘
void initBoard() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
board[i][j] = '-';
}
}
}
// 绘制棋盘
void drawBoard() {
for (int i = 1; i < 3; i++) {
line(CELL_SIZE * i, 0, CELL_SIZE * i, height);
line(0, CELL_SIZE * i, width, CELL_SIZE * i);
}
}
// 绘制棋子
void drawPieces() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (board[i][j] == 'X') {
drawX(i, j);
} else if (board[i][j] == 'O') {
drawO(i, j);
}
}
}
}
// 绘制X
void drawX(int row, int col) {
int x = col * CELL_SIZE + CELL_SIZE / 2;
int y = row * CELL_SIZE + CELL_SIZE / 2;
line(x - CELL_SIZE / 3, y - CELL_SIZE / 3, x + CELL_SIZE / 3, y + CELL_SIZE / 3);
line(x - CELL_SIZE / 3, y + CELL_SIZE / 3, x + CELL_SIZE / 3, y - CELL_SIZE / 3);
}
// 绘制O
void drawO(int row, int col) {
int x = col * CELL_SIZE + CELL_SIZE / 2;
int y = row * CELL_SIZE + CELL_SIZE / 2;
ellipse(x, y, CELL_SIZE / 1.5, CELL_SIZE / 1.5);
}
// 检查是否获胜
void checkWin() {
char winner = '-';
// 检查行
for (int i = 0; i < 3; i++) {
if (board[i][0] != '-' && board[i][0] == board[i][1] && board[i][1] == board[i][2]) {
winner = board[i][0];
}
}
// 检查列
for (int i = 0; i < 3; i++) {
if (board[0][i] != '-' && board[0][i] == board[1][i] && board[1][i] == board[2][i]) {
winner = board[0][i];
}
}
// 检查对角线
if (board[0][0] != '-' && board[0][0] == board[1][1] && board[1][1] == board[2][2]) {
winner = board[0][0];
}
if (board[0][2] != '-' && board[0][2] == board[1][1] && board[1][1] == board[2][0]) {
winner = board[0][2];
}
// 显示获胜者
if (winner != '-') {
textSize(100);
fill(255, 0, 0);
text(winner, width / 2, height / 2);
noLoop();
}
}
// 点击事件
void mousePressed() {
if (currentPlayer == 'X') {
int row = mouseY / CELL_SIZE;
int col = mouseX / CELL_SIZE;
if (board[row][col] == '-') {
board[row][col] = currentPlayer;
currentPlayer = 'O';
}
}
}
```
这个程序会创建一个3x3的井字棋棋盘。玩家可以点击棋盘的格子来下棋,程序会检查是否有获胜者。如果有获胜者,程序会在屏幕上显示获胜者的棋子,并停止运行。
阅读全文
相关推荐
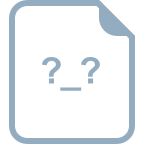
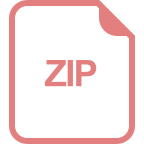
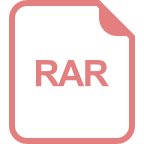















