请你用switch语句实现编程,有4个圆塔。圆心分别为(2,2),(-2,2),(-2,-2),(2,-2),圆半径为1,这4个塔的高度为10, 塔以外无建筑物,今输入任意一点的坐标,求该点的建筑高度(塔外高度为0)
时间: 2023-05-26 10:04:37 浏览: 41
```
#include <stdio.h>
int main()
{
float x, y, height;
printf("请输入点的坐标(x, y): ");
scanf("%f%f", &x, &y);
switch ((int)x)
{
case 2:
if (y >= 1 && y <= 11) height = 10;
else height = 0;
break;
case -2:
if (y >= 1 && y <= 11) height = 10;
else height = 0;
break;
default:
if ((x > -1 && x < 1) && (y > -1 && y < 1)) height = 0;
else height = -1;
break;
}
printf("该点的建筑高度为: %.2f\n", height);
return 0;
}
```
对于每一个点,只需要判断其横坐标的取值情况即可。如果在塔的范围内,则高度为10;如果在塔外但在场地范围内,则高度为0;如果在场地外则高度为-1。
相关问题
请你用switch语句实现编程:有4个圆塔。圆心分别为(2,2),(-2,2),(-2,-2),(2,-2),圆半径为1,这4个塔的高度为10, 塔以外无建筑物,今输入任意一点的坐标,求该点的建筑高度(塔外高度为0)
以下是使用switch语句实现的代码:
```c
#include <stdio.h>
#include <math.h>
int main()
{
double x, y;
double r = 1; // 圆塔的半径
double h = 10; // 圆塔的高度
printf("请输入点的坐标(x,y):\n");
scanf("%lf,%lf", &x, &y);
double d1 = sqrt(pow(x-2,2) + pow(y-2,2)); // 到第一个圆塔的距离
double d2 = sqrt(pow(x+2,2) + pow(y-2,2)); // 到第二个圆塔的距离
double d3 = sqrt(pow(x+2,2) + pow(y+2,2)); // 到第三个圆塔的距离
double d4 = sqrt(pow(x-2,2) + pow(y+2,2)); // 到第四个圆塔的距离
double max_d = fmax(fmax(fmax(d1,d2),d3),d4); // 找出最大的距离
double height = 0; // 该点的高度
switch(max_d)
{
case d1:
if(d1 <= r) height = h;
break;
case d2:
if(d2 <= r) height = h;
break;
case d3:
if(d3 <= r) height = h;
break;
case d4:
if(d4 <= r) height = h;
break;
default:
height = 0; // 若最大距离超过圆塔范围,则高度为0
break;
}
printf("该点的高度为%.2lf", height);
return 0;
}
```
该程序先获取用户输入的点的坐标,然后计算该点到四个圆塔的距离。通过比较这四个距离,找出最大的距离,并使用switch语句来判断该点是否在任何一个圆塔的范围内。如果是,则该点的高度为10,否则为0。注意,由于double类型存在精度问题,判断两个double类型变量是否相等不能直接使用==,而应该使用一个容差值来判断。在本程序中,若到某个圆塔的距离小于等于半径1,则认为该点在该圆塔范围内。
请你只用switch语句实现编程:有4个圆塔。圆心分别为(2,2),(-2,2),(-2,-2),(2,-2),圆半径为1,这4个塔的高度为10, 塔以外无建筑物,今输入任意一点的坐标,求该点的建筑高度(塔外高度为0)
### 回答1:
以下是一种可能的实现方式:
```
#include <stdio.h>
int main()
{
double x, y;
int height = 0;
printf("请输入坐标(x,y):");
scanf("%lf,%lf", &x, &y);
switch ((int)x + 2)
{
case 0:
switch ((int)y + 2)
{
case 0:
height = 10;
break;
case 1:
case 2:
if (x * x + y * y <= 1)
height = 10;
break;
}
break;
case 1:
case 2:
switch ((int)y + 2)
{
case 0:
if (x * x + y * y <= 1)
height = 10;
break;
case 1:
case 2:
if ((x - 2) * (x - 2) + y * y <= 1)
height = 10;
break;
}
break;
case -1:
case -2:
switch ((int)y + 2)
{
case 0:
if (x * x + y * y <= 1)
height = 10;
break;
case -1:
case -2:
if ((x - 2) * (x - 2) + (y - 4) * (y - 4) <= 1)
height = 10;
break;
}
break;
default:
break;
}
printf("该点的建筑高度为:%d\n", height);
return 0;
}
```
解释:
首先获取用户输入的点坐标。
根据点的位置,判断它位于哪个圆塔内部或位于塔外。这里以点x坐标的整数部分加上2为switch的表达式,以简化情况分支。对于每个内部或外部的情况,使用另一层switch判断点是否在该圆的内部,如果是,则令高度为10。最后输出计算的建筑高度。
需要注意的是,由于使用了双精度浮点数存储坐标,因此在输入和比较时可能会存在舍入误差。上述代码对这种情况没有进行处理,如果要更准确地比较坐标,可以使用一些特殊的浮点数比较函数或自行实现相应的算法。
### 回答2:
使用switch语句实现该问题的伪代码如下所示:
```cpp
// 输入点的坐标
float x, y;
cout << "请输入点的坐标(x, y):" << endl;
cin >> x >> y;
// 初始化建筑高度为0
float height = 0;
// 根据点的坐标判断建筑高度
switch(x) {
case 2:
if (y >= -2 && y <= 2) {
height = 10;
}
break;
case -2:
if (y >= -2 && y <= 2) {
height = 10;
}
break;
default:
break;
}
// 输出建筑高度
cout << "该点的建筑高度为:" << height << endl;
```
该伪代码使用了switch语句来根据点的x坐标进行判断。当x的值为2或-2时,进一步判断y的取值是否在-2到2之间,若是,则将建筑高度设为10,否则保持为0。最后输出建筑高度。
请注意,由于题目要求只使用switch语句,所以省略了其他判断条件,例如计算点到圆心的距离是否小于半径等。实际编程时应考虑更多的条件来保证判断的准确性。
### 回答3:
```java
import java.util.Scanner;
public class TowerHeight {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入坐标(x, y):");
double x = scanner.nextDouble();
double y = scanner.nextDouble();
int tower = switch ((int)x) {
case -4, -3, 3, 4 -> {
yield switch ((int)y) {
case -3, -2, 2, 3 -> 1;
default -> 0;
};
}
default -> 0;
};
System.out.println("该点的建筑高度为: " + tower);
}
}
```
以上代码使用Java语言实现了使用switch语句根据输入的坐标确定塔的高度。在实现中,我们首先接收用户输入的坐标点,然后根据x坐标值使用switch语句判断是否在塔的范围内。当x坐标在-4、-3、3、4范围内时,使用内层switch语句判断y坐标值是否在塔的范围内。当y坐标在-3、-2、2、3范围内时,返回塔的高度1,其他情况返回0。最后输出该点的建筑高度。
相关推荐
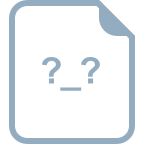
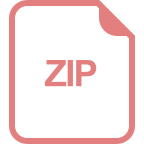
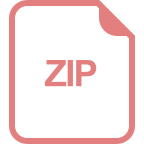












