根据Excel表格的A列的文本内容更新数据库表keywords_table中keyword列的值,python代码,sqlserver
时间: 2023-02-12 15:54:47 浏览: 77
可以使用 Python 的 pyodbc 库来连接 SQL Server 数据库,并使用 pandas 库读取 Excel 表格中的数据。
示例代码如下:
```python
import pyodbc
import pandas as pd
# 连接数据库
cnxn = pyodbc.connect('Driver={SQL Server};'
'Server=server_name;'
'Database=db_name;'
'Trusted_Connection=yes;')
# 读取 Excel 表格
df = pd.read_excel('file.xlsx', sheet_name='Sheet1', usecols='A')
# 遍历表格中的数据并更新数据库
for index, row in df.iterrows():
cursor = cnxn.cursor()
cursor.execute(f"UPDATE keywords_table SET keyword = '{row['A']}' WHERE id = {index + 1}")
cnxn.commit()
# 关闭连接
cnxn.close()
```
注意:在上面的示例代码中,需要替换 'server_name' 和 'db_name' 为实际的服务器名称和数据库名称。
相关问题
根据Excel表格的A列内容更新数据库表keywords_table中keyword列的值,python代码,sqlserver
首先,需要使用Python的pandas库读取Excel表格,然后使用pyodbc连接到SQL Server数据库。
具体代码如下:
```python
import pandas as pd
import pyodbc
# 读取Excel表格
df = pd.read_excel('path/to/excel.xlsx', usecols=['A'])
# 连接到SQL Server数据库
cnxn = pyodbc.connect('DRIVER={ODBC Driver 17 for SQL Server};'
'SERVER=your_server;'
'DATABASE=your_database;'
'UID=your_username;'
'PWD=your_password')
cursor = cnxn.cursor()
# 遍历Excel表格中的A列,执行更新操作
for i, row in df.iterrows():
cursor.execute("UPDATE keywords_table SET keyword=? WHERE id=?",
row['A'], i+1)
cnxn.commit()
# 关闭数据库连接
cursor.close()
cnxn.close()
```
需要注意的是,在这段代码中,假设Excel表格中A列数据对应着keywords_table中id列,并且这两列数据是一一对应的。如果实际情况不同,需要调整代码中的SQL语句。
根据Excel表格的列的文本内容更新数据库表keywords_table中keyword列的值,python代码,sqlserver
下面是一个示例代码,可以帮助你在 Python 中使用 SQL Server 更新 keywords_table 表中 keyword 列的值:
```
import pyodbc
import pandas as pd
# 连接到数据库
cnxn = pyodbc.connect('DRIVER={SQL Server};SERVER=server_name;DATABASE=db_name;UID=user_name;PWD=password')
cursor = cnxn.cursor()
# 读取 Excel 表格中的数据
df = pd.read_excel('path_to_excel_file.xlsx')
# 遍历行并更新 keywords_table 中的 keyword 列
for index, row in df.iterrows():
cursor.execute("UPDATE keywords_table SET keyword = ? WHERE id = ?", row['keyword'], row['id'])
cnxn.commit()
# 关闭数据库连接
cursor.close()
cnxn.close()
```
需要注意的是,这是一个示例代码,需要根据你的数据库和表结构进行相应的修改。
- 'server_name' 替换为你的服务器名
- 'db_name' 替换为你的数据库名
- 'user_name' 和 'password' 替换为你的用户名和密码
- 'path_to_excel_file.xlsx' 替换为 Excel 文件的完整路径
- 'keywords_table' 和 'keyword' 替换为你的表名和列名
相关推荐
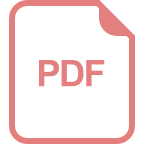






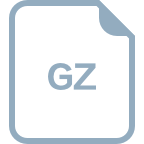
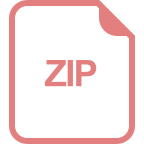
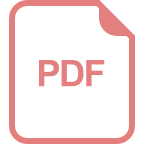
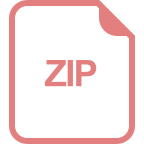
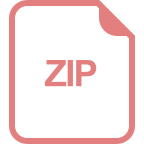
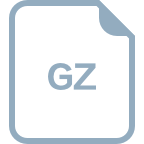