python自己编写代码实现hough变换
时间: 2024-01-01 15:04:57 浏览: 126
好的,下面是一个简单的 Python 代码实现 Hough 变换:
```python
import cv2
import numpy as np
import math
# 读取图像
img = cv2.imread('test.jpg', cv2.IMREAD_GRAYSCALE)
h, w = img.shape
# Canny 边缘检测
edges = cv2.Canny(img, 50, 150, apertureSize=3)
# Hough 变换
theta_res = 1
rho_res = 1
theta_range = int(180 / theta_res)
rho_max = int(math.sqrt(h ** 2 + w ** 2) / rho_res)
hough_acc = np.zeros((rho_max, theta_range), dtype=np.uint32)
for y in range(h):
for x in range(w):
if edges[y, x] > 0:
for itheta in range(theta_range):
theta = itheta * theta_res * math.pi / 180
rho = int(x * math.cos(theta) + y * math.sin(theta)) / rho_res
hough_acc[int(rho), itheta] += 1
# 阈值处理
threshold = 100
lines = []
for itheta in range(theta_range):
for irho in range(rho_max):
if hough_acc[irho, itheta] > threshold:
a = math.cos(itheta * theta_res * math.pi / 180)
b = math.sin(itheta * theta_res * math.pi / 180)
x0 = a * irho * rho_res
y0 = b * irho * rho_res
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
lines.append(((x1, y1), (x2, y2)))
# 绘制直线
for pt1, pt2 in lines:
cv2.line(img, pt1, pt2, (0, 0, 255), 2)
# 显示结果
cv2.imshow('Hough', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个代码实现了图像的 Canny 边缘检测,然后对边缘进行 Hough 变换,找到直线。最后将直线绘制在原图像上并显示出来。
阅读全文
相关推荐
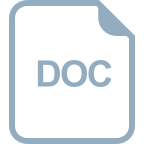
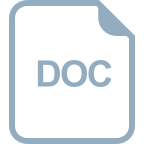
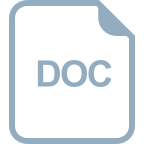








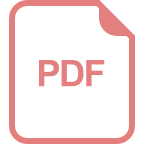
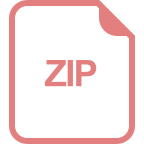
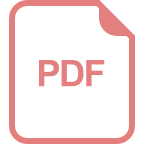
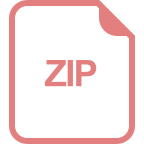
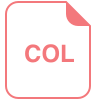

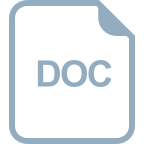
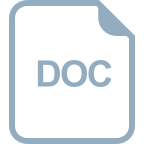