d3d12 渲染超过最大纹理大小的bmp图片代码
时间: 2024-03-19 12:44:55 浏览: 114
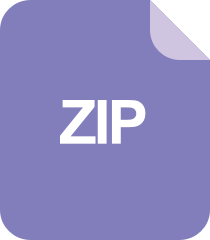
Kaguya:D3D12渲染引擎
如果你的图片超过了D3D12所支持的最大纹理大小,你可以使用以下代码来处理:
首先,将你的BMP图片加载到内存中。你可以使用GDI+或者DirectX Toolkit等工具来进行加载。
然后,将其分成多个小块,并将每个小块绘制到一个纹理上。你可以使用D3D12中的多个小纹理来模拟一个大纹理。
接下来,将小纹理拼接成一个大纹理。你可以使用纹理坐标来将小纹理拼接在一起。
最后,将大纹理绘制到屏幕上。
下面是一个简单的示例代码,用于将一个超过D3D12最大纹理大小的BMP图片绘制到屏幕上:
```C++
// Load the BMP image into memory
// ...
// Divide the image into small chunks and draw each chunk to a texture
const UINT ChunkSize = 2048; // The size of each chunk
const UINT ImageWidth = ...; // The width of the image
const UINT ImageHeight = ...; // The height of the image
std::vector<ID3D12Resource*> pTextureChunks;
for (UINT y = 0; y < ImageHeight; y += ChunkSize)
{
for (UINT x = 0; x < ImageWidth; x += ChunkSize)
{
// Create a texture for this chunk
CD3DX12_RESOURCE_DESC TextureDesc = CD3DX12_RESOURCE_DESC::Tex2D(
DXGI_FORMAT_R8G8B8A8_UNORM,
ChunkSize,
ChunkSize,
1, // Number of mip levels
1 // Number of samples
);
ID3D12Resource* pTextureChunk;
ThrowIfFailed(pDevice->CreateCommittedResource(
&CD3DX12_HEAP_PROPERTIES(D3D12_HEAP_TYPE_DEFAULT),
D3D12_HEAP_FLAG_NONE,
&TextureDesc,
D3D12_RESOURCE_STATE_COPY_DEST,
nullptr,
IID_PPV_ARGS(&pTextureChunk)));
// Copy the chunk of the image to the texture
D3D12_SUBRESOURCE_DATA TextureData = {};
// Calculate the size of the chunk
UINT ChunkWidth = min(ChunkSize, ImageWidth - x);
UINT ChunkHeight = min(ChunkSize, ImageHeight - y);
// Calculate the offset of the chunk in the image
UINT ImageOffsetX = x;
UINT ImageOffsetY = y;
// Calculate the offset of the chunk in the texture
UINT TextureOffsetX = 0;
UINT TextureOffsetY = 0;
// Set the source data
TextureData.pData = ...; // Pointer to the chunk of the image data
TextureData.RowPitch = ...; // The width of the chunk in bytes
TextureData.SlicePitch = ...; // The size of the chunk in bytes
// Copy the data to the texture
UpdateSubresources(
pCommandList,
pTextureChunk,
pTextureChunkUpload,
0, // The start subresource index
0, // The number of subresources to copy
1, // The number of texture chunks
&TextureData);
// Add the texture chunk to the list
pTextureChunks.push_back(pTextureChunk);
}
}
// Combine the texture chunks into a single texture
CD3DX12_RESOURCE_DESC CombinedTextureDesc = CD3DX12_RESOURCE_DESC::Tex2D(
DXGI_FORMAT_R8G8B8A8_UNORM,
ImageWidth,
ImageHeight,
1, // Number of mip levels
1 // Number of samples
);
ID3D12Resource* pCombinedTexture;
ThrowIfFailed(pDevice->CreateCommittedResource(
&CD3DX12_HEAP_PROPERTIES(D3D12_HEAP_TYPE_DEFAULT),
D3D12_HEAP_FLAG_NONE,
&CombinedTextureDesc,
D3D12_RESOURCE_STATE_COPY_DEST,
nullptr,
IID_PPV_ARGS(&pCombinedTexture)));
// Create a texture sampler
D3D12_SAMPLER_DESC SamplerDesc = {};
SamplerDesc.Filter = D3D12_FILTER_MIN_MAG_MIP_LINEAR;
SamplerDesc.AddressU = D3D12_TEXTURE_ADDRESS_MODE_WRAP;
SamplerDesc.AddressV = D3D12_TEXTURE_ADDRESS_MODE_WRAP;
SamplerDesc.AddressW = D3D12_TEXTURE_ADDRESS_MODE_WRAP;
SamplerDesc.MipLODBias = 0;
SamplerDesc.MaxAnisotropy = 1;
SamplerDesc.ComparisonFunc = D3D12_COMPARISON_FUNC_ALWAYS;
SamplerDesc.BorderColor[0] = 0.0f;
SamplerDesc.BorderColor[1] = 0.0f;
SamplerDesc.BorderColor[2] = 0.0f;
SamplerDesc.BorderColor[3] = 0.0f;
SamplerDesc.MinLOD = 0.0f;
SamplerDesc.MaxLOD = D3D12_FLOAT32_MAX;
CD3DX12_CPU_DESCRIPTOR_HANDLE SamplerHandle(pSamplerHeap->GetCPUDescriptorHandleForHeapStart());
pDevice->CreateSampler(&SamplerDesc, SamplerHandle);
// Create a shader resource view for the combined texture
D3D12_SHADER_RESOURCE_VIEW_DESC SRVDesc = {};
SRVDesc.Format = DXGI_FORMAT_R8G8B8A8_UNORM;
SRVDesc.ViewDimension = D3D12_SRV_DIMENSION_TEXTURE2D;
SRVDesc.Texture2D.MipLevels = 1;
SRVDesc.Texture2D.MostDetailedMip = 0;
CD3DX12_CPU_DESCRIPTOR_HANDLE SRVHandle(pSRVHeap->GetCPUDescriptorHandleForHeapStart());
pDevice->CreateShaderResourceView(pCombinedTexture, &SRVDesc, SRVHandle);
// Combine the texture chunks into the combined texture
for (UINT y = 0; y < ImageHeight; y += ChunkSize)
{
for (UINT x = 0; x < ImageWidth; x += ChunkSize)
{
// Find the texture chunk that contains this part of the image
UINT ChunkIndex = (y / ChunkSize) * (ImageWidth / ChunkSize) + (x / ChunkSize);
ID3D12Resource* pTextureChunk = pTextureChunks[ChunkIndex];
// Calculate the offset of the chunk in the image
UINT ImageOffsetX = x;
UINT ImageOffsetY = y;
// Calculate the offset of the chunk in the texture
UINT TextureOffsetX = x;
UINT TextureOffsetY = y;
// Copy the chunk to the combined texture
CD3DX12_TEXTURE_COPY_LOCATION Src(pTextureChunk, 0);
CD3DX12_TEXTURE_COPY_LOCATION Dst(pCombinedTexture, D3D12_SUBRESOURCE_INDEX_UNKNOWN, TextureOffsetX, TextureOffsetY);
pCommandList->CopyTextureRegion(&Dst, 0, 0, 0, &Src, nullptr);
}
}
// Draw the combined texture to the screen
// ...
```
请注意,这只是一个简单的示例代码,实际场景中可能需要根据具体情况进行调整。
阅读全文
相关推荐
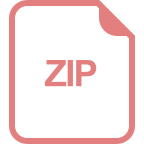















