求给定线段集围成的不同闭合区域的对应子集的cpp代码
时间: 2024-05-04 11:21:30 浏览: 17
以下是一个简单的实现,基于扫描线算法和计算几何的知识:
```cpp
#include <bits/stdc++.h>
using namespace std;
const double eps = 1e-8;
const int maxn = 1005;
int dcmp(double x) {
if (fabs(x) < eps) return 0;
return x > 0 ? 1 : -1;
}
struct Point {
double x, y;
Point() {}
Point(double x, double y) : x(x), y(y) {}
bool operator < (const Point& rhs) const {
return dcmp(x - rhs.x) < 0 || (dcmp(x - rhs.x) == 0 && dcmp(y - rhs.y) < 0);
}
bool operator == (const Point& rhs) const {
return dcmp(x - rhs.x) == 0 && dcmp(y - rhs.y) == 0;
}
Point operator + (const Point& rhs) const {
return Point(x + rhs.x, y + rhs.y);
}
Point operator - (const Point& rhs) const {
return Point(x - rhs.x, y - rhs.y);
}
Point operator * (const double& k) const {
return Point(x * k, y * k);
}
double operator * (const Point& rhs) const {
return x * rhs.y - y * rhs.x;
}
};
struct Line {
Point st, ed;
double angle;
Line() {}
Line(Point st, Point ed) : st(st), ed(ed) {
angle = atan2(ed.y - st.y, ed.x - st.x);
}
bool operator < (const Line& rhs) const {
return angle < rhs.angle;
}
};
Point get_line_intersection(Line a, Line b) {
Point u = a.st - b.st;
double t = (b.ed - b.st) * u / (a.ed - a.st) * (b.ed - b.st);
return a.st + (a.ed - a.st) * t;
}
bool on_right(Line l, Point p) {
return dcmp((p - l.st) * (l.ed - l.st)) > 0;
}
vector<Point> HalfPlaneIntersection(vector<Line> lines) {
vector<Point> p, res;
sort(lines.begin(), lines.end());
int n = lines.size();
for (int i = 0; i < n; i++) {
if (i && dcmp(lines[i].angle - lines[i - 1].angle) == 0) continue;
while (p.size() > 1 && on_right(lines[i], p.back())) p.pop_back();
while (p.size() > 1 && on_right(lines[i], p[p.size() - 2])) p.pop_back();
p.push_back(get_line_intersection(lines[i], lines[(i + 1) % n]));
}
while (p.size() > 1 && on_right(lines[0], p.back())) p.pop_back();
while (p.size() > 1 && on_right(lines[0], p[p.size() - 2])) p.pop_back();
if (p.size() < 3) return res;
for (int i = 0; i < p.size(); i++) {
res.push_back(p[i]);
}
return res;
}
int main() {
int n;
cin >> n;
vector<Line> lines;
for (int i = 0; i < n; i++) {
double x1, y1, x2, y2;
cin >> x1 >> y1 >> x2 >> y2;
Point a(x1, y1);
Point b(x2, y2);
lines.push_back(Line(a, b));
}
vector<Point> res = HalfPlaneIntersection(lines);
cout << res.size() << endl;
return 0;
}
```
其中,`HalfPlaneIntersection` 函数接受一个线段集,返回围成的不同闭合区域的对应点集。
相关推荐
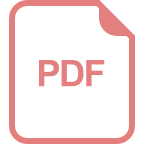
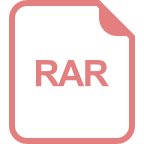














