定义表示代数多项式中一项的结构体struct Item类型如下: double coeff;//多项式系数 int power;//x的幂 在主函数main()中定义struct Item类型变量item1,在此基础上。为结构体struct Item类型增加struct Item* next成员。并以此为结点,使用malloc函数动态建立多项式单链表。程序结束前使用free函数释放单链表所有结点。
时间: 2024-02-19 21:57:13 浏览: 82
好的,让我来帮你解答这个问题。
首先,我们需要定义一个结构体 `Item` 来表示代数多项式中的每一项,包括它的系数和指数。代码如下:
```c
struct Item {
double coeff; // 多项式系数
int power; // x 的幂
struct Item* next; // 指向下一项的指针
};
```
接下来,在 `main` 函数中,我们可以定义一个 `Item` 类型的变量 `item1`,代码如下:
```c
struct Item item1;
item1.coeff = 1.0;
item1.power = 2;
item1.next = NULL;
```
然后,我们需要使用 `malloc` 函数动态分配内存来建立多项式单链表。这里我们可以编写一个函数 `createList` 来实现这个过程。代码如下:
```c
struct Item* createList() {
struct Item* head = NULL;
struct Item* tail = NULL;
int n;
printf("请输入多项式的项数:");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("请输入第 %d 项的系数和指数:", i + 1);
double coeff;
int power;
scanf("%lf %d", &coeff, &power);
struct Item* node = (struct Item*) malloc(sizeof(struct Item));
node->coeff = coeff;
node->power = power;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
```
这个函数会提示用户输入多项式的项数,然后逐一读入每一项的系数和指数,并将它们存储在一个新的 `Item` 结构体中。最后,将这个结构体加入到链表的末尾。
最后,我们需要使用 `free` 函数释放单链表的所有结点。这里我们可以编写一个函数 `destroyList` 来实现这个过程。代码如下:
```c
void destroyList(struct Item* head) {
struct Item* p = head;
while (p != NULL) {
struct Item* q = p;
p = p->next;
free(q);
}
}
```
这个函数会从链表的头结点开始,逐一遍历链表中的每个结点,并释放它们占用的内存。
完整的程序代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
struct Item {
double coeff; // 多项式系数
int power; // x 的幂
struct Item* next; // 指向下一项的指针
};
struct Item* createList() {
struct Item* head = NULL;
struct Item* tail = NULL;
int n;
printf("请输入多项式的项数:");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("请输入第 %d 项的系数和指数:", i + 1);
double coeff;
int power;
scanf("%lf %d", &coeff, &power);
struct Item* node = (struct Item*) malloc(sizeof(struct Item));
node->coeff = coeff;
node->power = power;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
void destroyList(struct Item* head) {
struct Item* p = head;
while (p != NULL) {
struct Item* q = p;
p = p->next;
free(q);
}
}
int main() {
struct Item item1;
item1.coeff = 1.0;
item1.power = 2;
item1.next = NULL;
struct Item* head = createList();
destroyList(head);
return 0;
}
```
阅读全文
相关推荐
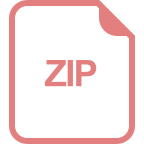












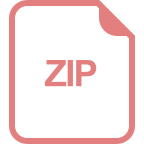
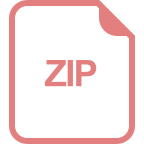