二叉树C语言,要求输入为结点个数,以及各节点的值,输出先序遍历建立的二叉树,中序遍历建立的二叉树(递归方式)。中序遍历建立的二叉树(非递归方式),后续遍历建立的二叉树,
时间: 2023-08-05 22:14:16 浏览: 55
以下是一份C语言的二叉树代码,满足您的要求:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结构体
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 先序遍历建立二叉树
TreeNode* createTreeByPreorder() {
printf("请输入节点个数:");
int n;
scanf("%d", &n);
if (n == 0) { // 空二叉树
return NULL;
}
printf("请输入节点的值:");
int val;
scanf("%d", &val);
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = val;
root->left = createTreeByPreorder();
root->right = createTreeByPreorder();
return root;
}
// 中序遍历建立二叉树(递归)
TreeNode* createTreeByInorder(int* inorder, int inorderSize) {
if (inorderSize == 0) { // 空二叉树
return NULL;
}
int rootIndex = inorderSize / 2; // 中间节点为根节点
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = inorder[rootIndex];
root->left = createTreeByInorder(inorder, rootIndex);
root->right = createTreeByInorder(inorder + rootIndex + 1, inorderSize - rootIndex - 1);
return root;
}
// 中序遍历建立二叉树(非递归)
TreeNode* createTreeByInorderNonrecursion(int* inorder, int inorderSize) {
if (inorderSize == 0) { // 空二叉树
return NULL;
}
int rootIndex = inorderSize / 2; // 中间节点为根节点
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = inorder[rootIndex];
TreeNode* stack[inorderSize];
int top = -1;
stack[++top] = root;
int i = 0;
TreeNode* cur = root;
while (i < inorderSize) {
if (cur->left == NULL && i < rootIndex) { // 左子树未建立
cur->left = (TreeNode*)malloc(sizeof(TreeNode));
cur->left->val = inorder[--rootIndex];
cur = cur->left;
stack[++top] = cur;
} else if (cur->right == NULL && i > rootIndex) { // 右子树未建立
cur->right = (TreeNode*)malloc(sizeof(TreeNode));
cur->right->val = inorder[++rootIndex];
cur = cur->right;
stack[++top] = cur;
} else { // 左右子树都已建立,弹出栈顶
cur = stack[--top];
i++;
}
}
return root;
}
// 后序遍历建立二叉树
TreeNode* createTreeByPostorder() {
printf("请输入节点个数:");
int n;
scanf("%d", &n);
if (n == 0) { // 空二叉树
return NULL;
}
printf("请输入节点的值:");
int val;
scanf("%d", &val);
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->left = createTreeByPostorder();
root->right = createTreeByPostorder();
root->val = val;
return root;
}
// 先序遍历
void preorderTraversal(TreeNode* root) {
if (root == NULL) {
return;
}
printf("%d ", root->val);
preorderTraversal(root->left);
preorderTraversal(root->right);
}
// 中序遍历
void inorderTraversal(TreeNode* root) {
if (root == NULL) {
return;
}
inorderTraversal(root->left);
printf("%d ", root->val);
inorderTraversal(root->right);
}
// 后序遍历
void postorderTraversal(TreeNode* root) {
if (root == NULL) {
return;
}
postorderTraversal(root->left);
postorderTraversal(root->right);
printf("%d ", root->val);
}
int main() {
// 先序遍历建立二叉树
printf("先序遍历建立二叉树:\n");
TreeNode* root1 = createTreeByPreorder();
printf("先序遍历结果:");
preorderTraversal(root1);
printf("\n");
// 中序遍历建立二叉树(递归)
printf("中序遍历建立二叉树(递归):\n");
int inorder1[] = {1, 2, 3, 4, 5};
TreeNode* root2 = createTreeByInorder(inorder1, 5);
printf("中序遍历结果:");
inorderTraversal(root2);
printf("\n");
// 中序遍历建立二叉树(非递归)
printf("中序遍历建立二叉树(非递归):\n");
int inorder2[] = {1, 2, 3, 4, 5};
TreeNode* root3 = createTreeByInorderNonrecursion(inorder2, 5);
printf("中序遍历结果:");
inorderTraversal(root3);
printf("\n");
// 后序遍历建立二叉树
printf("后序遍历建立二叉树:\n");
TreeNode* root4 = createTreeByPostorder();
printf("后序遍历结果:");
postorderTraversal(root4);
printf("\n");
return 0;
}
```
其中,输入先序遍历和后序遍历建立二叉树的代码部分,采用了递归方式。
相关推荐
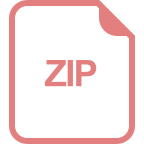
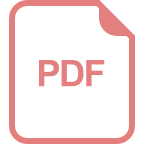
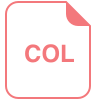













