编写并调试一个模拟的进程调度程序,采用“最高优先数优先”调度算法对五个进程进行调度。
时间: 2023-05-25 13:03:06 浏览: 203
以下是一个基于 Python 的简单模拟进程调度程序,采用“最高优先数优先”(Highest Priority First,HPF)调度算法对五个进程进行调度。在此示例中,所有进程都具有相同的执行时间,但有不同的优先级。高优先级的进程将比低优先级的进程更快地得到 CPU 时间片,如果有多个进程具有相同的最高优先级,则将选择先进先出(FIFO)顺序。
```python
import queue
# Define process class
class Process:
def __init__(self, pid, priority):
self.pid = pid
self.priority = priority
def __repr__(self):
return f"Process: {self.pid} | Priority: {self.priority}"
# Define HPF scheduling algorithm function
def hpf_scheduling(processes):
ready_queue = queue.PriorityQueue()
# Add processes to ready queue
for p in processes:
ready_queue.put((-p.priority, p)) # Prioritize by negative priority
# Run processes in ready queue
while not ready_queue.empty():
priority, p = ready_queue.get()
print(f"Processing: {p} | Remaining processes: {ready_queue.qsize()}")
# Simulate running process for some time
for i in range(10000000):
pass
# Define sample processes
processes = [
Process(1, 2),
Process(2, 1),
Process(3, 3),
Process(4, 2),
Process(5, 3)
]
# Run HPF scheduling algorithm on processes
hpf_scheduling(processes)
```
在上述代码中,我们首先定义了进程类,具有进程 ID 和优先级属性。然后,我们定义了 HPF 调度算法函数,该函数接受进程列表并按其优先级添加到优先级队列中。我们使用 Python 的内置优先级队列(`PriorityQueue`)来实现这个优先级队列。然后,我们从就绪队列中持续地弹出实现了最高优先级并按 FIFO 顺序的所有进程,并模拟了运行进程的一些时间。最后,我们定义了一个样例进程列表并调用 HPF 调度算法函数。
在本示例中,我们针对五个具有不同优先级的进程进行模拟调度。您可以尝试更改进程的优先级,添加更多进程,通过模拟 CPU 时间片中断等实现其他更高级别的功能以扩展此程序。
阅读全文
相关推荐
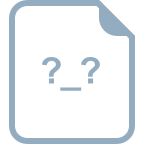





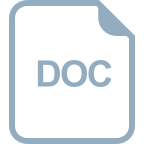
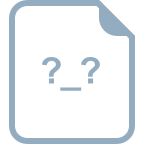







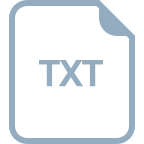