cv2.normalize()中的src和dst是什么关系
时间: 2024-05-20 12:10:49 浏览: 14
在OpenCV中,cv2.normalize()函数用于归一化数组。src参数是输入数组,dst参数是输出数组。如果dst参数为None,则函数会在原始数组上进行归一化操作。如果dst参数不为None,则函数会将归一化结果存储到输出数组中。可以通过设置norm_type参数来指定归一化类型。如果指定了norm_type为cv2.NORM_INF,则函数将使用L∞范数进行归一化。如果指定了norm_type为cv2.NORM_L1,则函数将使用L1范数进行归一化。如果指定了norm_type为cv2.NORM_L2,则函数将使用L2范数进行归一化。此外,可以通过设置alpha和beta参数来指定归一化范围。例如,如果设置alpha为0和beta为1,则函数将对数组进行归一化,使得数组中的最小值为0,最大值为1。
相关问题
cv2.normalize 参数要输入什么
cv2.normalize函数用于将输入数组归一化到指定范围内。它的参数包括:
- src:输入数组
- dst:输出数组,与src大小和类型相同
- alpha:归一化范围的最小值
- beta:归一化范围的最大值
- norm_type:归一化类型,包括cv2.NORM_INF,cv2.NORM_L1,cv2.NORM_L2等,默认为cv2.NORM_L2
- dtype:输出数组的数据类型,默认为与src相同的数据类型
例如,要将输入数组归一化到0到255的范围内,可以这样调用cv2.normalize函数:
```
cv2.normalize(src, dst, 0, 255, cv2.NORM_MINMAX)
```
cv2.normalize参数详解
cv2.normalize函数是OpenCV中用于归一化数组的函数。它的语法如下:
```python
dst = cv2.normalize(src, dst, alpha, beta, norm_type, dtype, mask)
```
其中,参数的含义如下:
- src:输入数组。
- dst:输出数组,与src大小和类型相同。
- alpha:归一化范围的最小值。
- beta:归一化范围的最大值。
- norm_type:归一化类型,包括cv2.NORM_INF、cv2.NORM_L1、cv2.NORM_L2、cv2.NORM_MINMAX等。
- dtype:输出数组的数据类型。
- mask:掩膜数组。
举个例子,如果我们想将一个灰度图像归一化到0到255的范围内,可以使用以下代码:
```python
import cv2
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
dst = cv2.normalize(img, None, 0, 255, cv2.NORM_MINMAX)
```
这样,dst就是归一化后的图像。
相关推荐
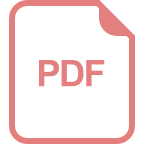
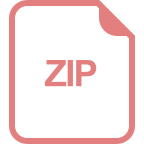












