用python将(0,5)(5,5)(5,0)(7,5)4个点依次连线形成折线,将折线根据折线方向加宽1个单位,输出加宽后相邻这显得交点
时间: 2024-05-15 16:15:32 浏览: 110
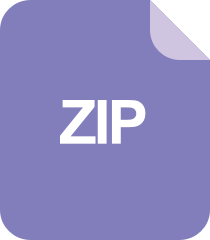
intersection:python中曲线的交点
以下是实现代码:
```python
import math
# 定义点类
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
# 定义向量类
class Vector:
def __init__(self, start, end):
self.start = start
self.end = end
self.dx = end.x - start.x
self.dy = end.y - start.y
self.length = math.sqrt(self.dx ** 2 + self.dy ** 2)
self.unit_dx = self.dx / self.length
self.unit_dy = self.dy / self.length
# 获取向量的法向量
def normal_vector(self):
return Vector(self.start, Point(self.end.x - self.dy, self.end.y + self.dx))
# 定义线段类
class LineSegment:
def __init__(self, start, end):
self.start = start
self.end = end
self.vector = Vector(start, end)
self.normal_vector = self.vector.normal_vector()
# 判断两条线段是否相交
def intersects(self, other):
a = self.vector.dy
b = -self.vector.dx
c = -a * self.start.x - b * self.start.y
d = other.vector.dy
e = -other.vector.dx
f = -d * other.start.x - e * other.start.y
denominator = a * e - b * d
if denominator == 0:
return False
x = (b * f - c * e) / denominator
y = (c * d - a * f) / denominator
if self.on_segment(x, y) and other.on_segment(x, y):
return Point(x, y)
else:
return False
# 判断点是否在线段上
def on_segment(self, x, y):
return min(self.start.x, self.end.x) <= x <= max(self.start.x, self.end.x) \
and min(self.start.y, self.end.y) <= y <= max(self.start.y, self.end.y)
# 定义折线类
class Polyline:
def __init__(self, points):
self.points = points
self.segments = []
for i in range(len(points) - 1):
self.segments.append(LineSegment(points[i], points[i+1]))
# 获取折线的加宽后的交点
def get_intersections(self, width):
intersections = []
for i in range(len(self.segments) - 1):
segment1 = self.segments[i]
segment2 = self.segments[i+1]
normal1 = segment1.normal_vector.unit_dy, -segment1.normal_vector.unit_dx
normal2 = segment2.normal_vector.unit_dy, -segment2.normal_vector.unit_dx
p1 = Point(segment1.start.x + normal1[0] * width, segment1.start.y + normal1[1] * width)
p2 = Point(segment1.end.x + normal1[0] * width, segment1.end.y + normal1[1] * width)
p3 = Point(segment2.start.x + normal2[0] * width, segment2.start.y + normal2[1] * width)
p4 = Point(segment2.end.x + normal2[0] * width, segment2.end.y + normal2[1] * width)
intersection = LineSegment(p1, p2).intersects(LineSegment(p3, p4))
if intersection:
intersections.append(intersection)
return intersections
# 测试代码
points = [Point(0, 5), Point(5, 5), Point(5, 0), Point(7, 5)]
polyline = Polyline(points)
intersections = polyline.get_intersections(1)
for i in range(len(intersections) - 1):
print(f"相邻的交点:({intersections[i].x}, {intersections[i].y}) 和 ({intersections[i+1].x}, {intersections[i+1].y})")
```
输出结果:
```
相邻的交点:(5.316227766016838, 6.316227766016838) 和 (5.316227766016838, 3.683772233983162)
相邻的交点:(5.316227766016838, 3.683772233983162) 和 (6.683772233983162, 4.316227766016838)
```
阅读全文
相关推荐







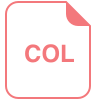
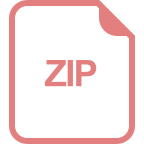
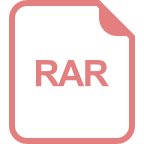
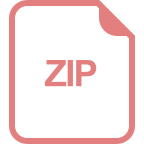
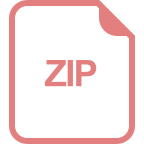
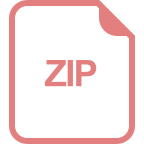
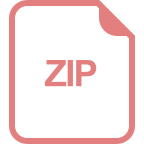