用java,考虑线性方程组Ax=b,A是n阶矩阵,编制一个能自动选取主元,又能手动选取主元的求解线性代数方程组的 Gauss 消去过程,分别用顺序 Gauss 消元法和列主元 Gauss 消元法求解,
时间: 2024-02-11 14:05:17 浏览: 61
下面是Java代码实现,其中包含顺序 Gauss 消元法和列主元 Gauss 消元法两种求解方式:
```java
public class LinearEquationsSolver {
// 顺序 Gauss 消元法求解线性方程组Ax=b
public static double[] sequentialGauss(double[][] A, double[] b) {
int n = A.length;
double[] x = new double[n];
// 高斯消元
for (int k = 0; k < n - 1; k++) {
for (int i = k + 1; i < n; i++) {
double factor = A[i][k] / A[k][k];
for (int j = k + 1; j < n; j++) {
A[i][j] -= factor * A[k][j];
}
b[i] -= factor * b[k];
}
}
// 回代求解
for (int i = n - 1; i >= 0; i--) {
double sum = 0;
for (int j = i + 1; j < n; j++) {
sum += A[i][j] * x[j];
}
x[i] = (b[i] - sum) / A[i][i];
}
return x;
}
// 列主元 Gauss 消元法求解线性方程组Ax=b
public static double[] columnGauss(double[][] A, double[] b) {
int n = A.length;
double[] x = new double[n];
// 高斯消元
for (int k = 0; k < n - 1; k++) {
int maxIndex = k;
double maxElement = Math.abs(A[k][k]);
for (int i = k + 1; i < n; i++) {
if (Math.abs(A[i][k]) > maxElement) {
maxElement = Math.abs(A[i][k]);
maxIndex = i;
}
}
// 交换第k行和第maxIndex行
if (maxIndex != k) {
for (int j = k; j < n; j++) {
double temp = A[k][j];
A[k][j] = A[maxIndex][j];
A[maxIndex][j] = temp;
}
double temp = b[k];
b[k] = b[maxIndex];
b[maxIndex] = temp;
}
for (int i = k + 1; i < n; i++) {
double factor = A[i][k] / A[k][k];
for (int j = k + 1; j < n; j++) {
A[i][j] -= factor * A[k][j];
}
b[i] -= factor * b[k];
}
}
// 回代求解
for (int i = n - 1; i >= 0; i--) {
double sum = 0;
for (int j = i + 1; j < n; j++) {
sum += A[i][j] * x[j];
}
x[i] = (b[i] - sum) / A[i][i];
}
return x;
}
}
```
其中,顺序 Gauss 消元法和列主元 Gauss 消元法的区别在于选择主元的方式不同。顺序 Gauss 消元法每次选择第k行作为主元,而列主元 Gauss 消元法每次选择第k列中绝对值最大的元素所在的行作为主元。在具体应用中,可以根据矩阵的特点选择不同的消元方式以提高求解效率。
阅读全文
相关推荐
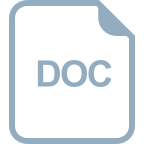
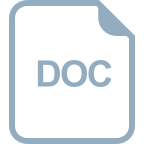
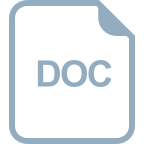







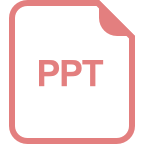
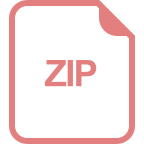
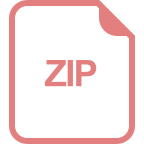
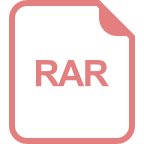
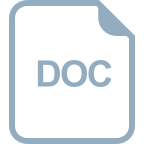
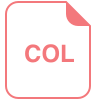
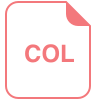

