用C++语言设计一个程序,写出满足以下要求的完整代码。定义一个时间类Time,能够提供和设置由时、分、秒组成的时间(设置为3时32分48秒),并编写出应用程序,定义时间对象,设置时间,输出该对象提供的时间(输出格式为:03:32:48)。
时间: 2023-05-13 13:06:07 浏览: 107
以下是满足要求的完整代码:
```
#include <stdio.h>
typedef struct {
int hour;
int minute;
int second;
} Time;
void setTime(Time *t, int hour, int minute, int second) {
t->hour = hour;
t->minute = minute;
t->second = second;
}
void printTime(Time t) {
printf("%02d:%02d:%02d\n", t.hour, t.minute, t.second);
}
int main() {
Time t;
setTime(&t, 3, 32, 48);
printTime(t);
return 0;
}
```
这个程序定义了一个时间类Time,包含时、分、秒三个成员变量。通过setTime函数可以设置时间,通过printTime函数可以输出时间。在主函数中,定义了一个时间对象t,设置为3时32分48秒,然后输出该对象提供的时间,输出格式为03:32:48。
相关问题
C++定义一个时间类,能提供和设置时分秒组成的时间,并写出应用程序、定义时间对象、设置时间、输出该对象提供的时间
#include <iostream>
using namespace std;
class Time {
private:
int hour;
int minute;
int second;
public:
void setTime(int h, int m, int s) {
hour = h;
minute = m;
second = s;
}
void getTime() {
cout << "Time is: " << hour << ":" << minute << ":" << second << endl;
}
};
int main() {
Time t1; //定义时间对象
t1.setTime(12, 30, 45); //设置时间
t1.getTime(); //输出该对象提供的时间
return 0;
}
用c++写一个程序,将24小时时间格式转化为12小时时间格式。定义一个time mistake的异常类,处理用户输入的非法时间,比如10:65或者无效字符
好的,我可以帮你写一个C++程序来实现这个功能,并且处理用户输入的非法时间。下面是代码,希望能够帮到你:
```cpp
#include <iostream>
#include <string>
#include <regex>
using namespace std;
class time_mistake : public exception {
public:
const char* what() const throw() {
return "非法时间格式!";
}
};
string convertTimeFormat(string time) {
regex pattern("([0-9]{2}):([0-9]{2})");
smatch match;
regex_search(time, match, pattern);
int hour = stoi(match[1]);
int minute = stoi(match[2]);
if (hour < 0 || hour > 23 || minute < 0 || minute > 59) {
throw time_mistake();
}
string suffix = hour < 12 ? "AM" : "PM";
hour = hour % 12;
if (hour == 0) {
hour = 12;
}
return to_string(hour) + ":" + match[2] + " " + suffix;
}
int main() {
string time;
cout << "请输入24小时时间格式(如:13:30):" << endl;
cin >> time;
try {
string result = convertTimeFormat(time);
cout << "12小时时间格式为:" << result << endl;
}
catch (time_mistake& e) {
cerr << "Error: " << e.what() << endl;
}
return 0;
}
```
程序中定义了一个`time_mistake`异常类,用来处理用户输入的非法时间。在`convertTimeFormat`函数中,使用正则表达式提取输入字符串中的小时和分钟,并进行格式转换。如果输入时间不符合规范,将抛出`time_mistake`异常。在`main`函数中,捕获异常并输出错误信息,或者输出转换后的时间格式。
希望这个程序能够满足你的需求,如果还有其他问题,请随时提出。
阅读全文
相关推荐
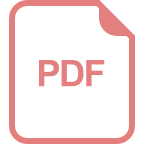












