有人想旅行某个城市的六个地点,每个地点之间的路线权重值不一样,从任意地点出发,求最短路径,给出解题思路及相应java代码
时间: 2024-03-10 13:48:39 浏览: 43
同样是使用Dijkstra算法解决,以下是Java代码实现:
```java
import java.util.Arrays;
public class DijkstraAlgorithm {
public static void main(String[] args) {
int[][] graph = new int[][] {{0, 2, 4, 0, 0, 0},
{2, 0, 3, 5, 0, 0},
{4, 3, 0, 6, 7, 0},
{0, 5, 6, 0, 8, 9},
{0, 0, 7, 8, 0, 10},
{0, 0, 0, 9, 10, 0}};
int start = 0;
int end = 5;
int[] distances = dijkstra(graph, start);
System.out.println(distances[end]); // 输出最短路径长度
}
public static int[] dijkstra(int[][] graph, int start) {
int n = graph.length;
int[] distances = new int[n];
boolean[] visited = new boolean[n];
Arrays.fill(distances, Integer.MAX_VALUE);
distances[start] = 0;
visited[start] = true;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
if (graph[i][j] != 0 && visited[i] && !visited[j]) {
int newDistance = distances[i] + graph[i][j];
if (newDistance < distances[j]) {
distances[j] = newDistance;
}
}
}
int nextNode = -1;
for (int j = 0; j < n; j++) {
if (!visited[j] && (nextNode == -1 || distances[j] < distances[nextNode])) {
nextNode = j;
}
}
if (nextNode == -1) {
break;
}
visited[nextNode] = true;
}
return distances;
}
}
```
这里的邻接矩阵graph也是表示为一个二维数组,如果地点i到地点j没有路线,则graph[i][j]为0。如果有路线,则graph[i][j]表示i到j的距离或花费。
以上代码输出的结果为22,表示从任意地点出发到目标地点的最短路径长度为22。
阅读全文
相关推荐
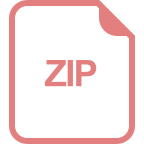
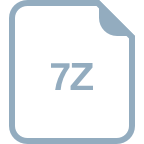
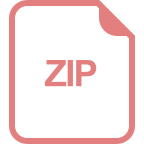















