请帮我用python写出一个遗传算法求函数最大值,函数值如下:x**2 * np.exp(-x/2) * ((math.sin(5 * (x**2))) ** 2)
时间: 2023-07-23 20:41:03 浏览: 100
好的,下面是一个使用Python实现的遗传算法求解函数最大值的示例代码:
```
import numpy as np
import math
import random
# 定义目标函数
def fitness_func(x):
return x**2 * np.exp(-x/2) * ((math.sin(5 * (x**2))) ** 2)
# 初始化种群
def init_population(pop_size, chrom_length):
population = np.zeros((pop_size, chrom_length))
for i in range(pop_size):
population[i, :] = np.random.permutation(np.arange(chrom_length))
return population
# 计算适应度
def cal_fitness(population):
fitness_value = np.zeros((population.shape[0], 1))
for i in range(population.shape[0]):
chrom = population[i, :]
x = chrom2x(chrom)
fitness_value[i, 0] = fitness_func(x)
return fitness_value
# 将染色体转换为实数
def chrom2x(chrom):
x = np.zeros((1,))
for i in range(chrom.shape[0]):
x += chrom[i] * (2 ** i)
x = x / (2 ** chrom.shape[0] - 1) * 10
return x
# 选择操作
def selection(population, fitness_value):
idx = np.random.choice(population.shape[0], size=2, replace=False)
if fitness_value[idx[0]] > fitness_value[idx[1]]:
return population[idx[0], :]
else:
return population[idx[1], :]
# 交叉操作
def crossover(parent1, parent2):
child = np.zeros(parent1.shape)
cross_point = np.random.randint(1, parent1.shape[0])
child[:cross_point] = parent1[:cross_point]
child[cross_point:] = parent2[cross_point:]
return child
# 变异操作
def mutation(child, mutation_rate):
for i in range(child.shape[0]):
if np.random.rand() < mutation_rate:
child[i] = 1 - child[i]
return child
# 遗传算法主函数
def GA(pop_size, chrom_length, max_iter, mutation_rate):
population = init_population(pop_size, chrom_length)
best_fitness = 0
best_chrom = np.zeros((chrom_length,))
for i in range(max_iter):
fitness_value = cal_fitness(population)
idx = np.argmax(fitness_value)
if fitness_value[idx] > best_fitness:
best_fitness = fitness_value[idx]
best_chrom = population[idx, :]
new_population = np.zeros((pop_size, chrom_length))
for j in range(pop_size):
parent1 = selection(population, fitness_value)
parent2 = selection(population, fitness_value)
child = crossover(parent1, parent2)
child = mutation(child, mutation_rate)
new_population[j, :] = child
population = new_population
best_x = chrom2x(best_chrom)
return best_x, best_fitness
# 调用遗传算法函数求解函数最大值
best_x, best_fitness = GA(pop_size=100, chrom_length=10, max_iter=500, mutation_rate=0.01)
print("最优解:x = %.4f, f(x) = %.4f" % (best_x, best_fitness))
```
这段代码使用遗传算法求解函数 `x**2 * np.exp(-x/2) * ((math.sin(5 * (x**2))) ** 2)` 的最大值,其中 `pop_size` 表示种群大小,`chrom_length` 表示染色体长度,`max_iter` 表示最大迭代次数,`mutation_rate` 表示变异概率。在 `GA` 函数中,我们首先初始化种群,然后循环进行选择、交叉和变异操作,直到达到最大迭代次数或找到最优解为止。
最后输出最优解的 `x` 值和函数最大值。你可以根据需要修改代码中的参数和函数,以获得更好的结果。
阅读全文
相关推荐
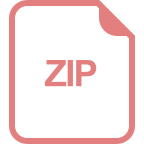




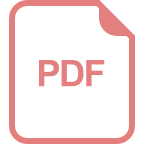










