我需要用python合并一个文件夹,并输出为csv格式的文件,代码怎么写呢
时间: 2023-03-11 09:48:44 浏览: 113
可以使用python的shutil模块来合并文件夹,下面是一个示例代码:import shutil
import os # 要合并的文件夹
src_folder = '/path/to/src/folder'
# 输出的csv文件
dst_csv = '/path/to/dst/csv' # 合并文件夹
shutil.make_archive(dst_csv, 'zip', src_folder)
# 解压缩文件
shutil.unpack_archive(dst_csv + '.zip', dst_csv, 'zip')
# 删除压缩文件
os.remove(dst_csv + '.zip')
相关问题
python合并同一个文件夹中的csv文件
您好,对于您的问题,我可以回答。您可以使用Python的pandas库来实现该功能。您可以使用pandas的concat函数将同一个文件夹下的多个csv文件进行合并。具体操作流程可以参考以下代码示例:
```python
import os
import pandas as pd
# 获取当前文件夹下所有的csv文件
csv_files = [f for f in os.listdir() if f.endswith('.csv')]
# 使用pandas的concat函数将所有csv文件合并成一个DataFrame对象
df = pd.concat([pd.read_csv(f) for f in csv_files])
# 将合并后的数据保存到一个新文件中
df.to_csv('merged_file.csv', index=False)
```
以上代码会先获取当前文件夹下所有的csv文件,然后使用pandas的concat函数将这些文件合并成一个DataFrame对象。最后,将合并后的数据保存到一个新的csv文件中。希望能对您有所帮助。
python合并文件夹下所有的csv文件代码
可以使用Python内置的csv模块和os模块来实现合并文件夹下所有的csv文件。具体代码如下:
```python
import csv
import os
folder_path = 'folder_path' # 文件夹路径
output_file = 'output.csv' # 合并后的文件名
with open(output_file, 'w', newline='') as outfile:
writer = csv.writer(outfile)
header_written = False
for dirpath, dirnames, filenames in os.walk(folder_path):
for file in filenames:
if file.endswith('.csv'):
file_path = os.path.join(dirpath, file)
with open(file_path, 'r') as infile:
reader = csv.reader(infile)
if not header_written:
header = next(reader)
writer.writerow(header)
header_written = True
for row in reader:
writer.writerow(row)
```
代码中,首先指定要合并的文件夹路径和合并后的文件名。然后使用`os.walk`函数遍历文件夹下的所有文件,如果是以`.csv`结尾的文件,就将其逐行读取并写入到输出文件中。在写入第一个文件时,将其表头也写入输出文件中。最终输出的文件即为合并后的文件。
阅读全文
相关推荐
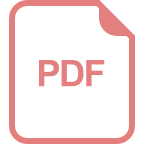



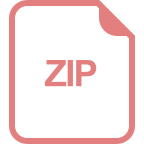
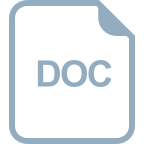








