eclipse milo 使用示例
时间: 2023-07-11 11:16:57 浏览: 330
好的,我会为您提供一个简单的Eclipse Milo使用示例。
在这个示例中,我们将使用Eclipse Milo创建一个OPC UA客户端,并从OPC UA服务器读取节点的值。以下是示例代码:
```java
import org.eclipse.milo.opcua.sdk.client.OpcUaClient;
import org.eclipse.milo.opcua.sdk.client.api.config.OpcUaClientConfig;
import org.eclipse.milo.opcua.sdk.client.api.identity.UsernameProvider;
import org.eclipse.milo.opcua.stack.core.security.SecurityPolicy;
import org.eclipse.milo.opcua.stack.core.types.builtin.DataValue;
import org.eclipse.milo.opcua.stack.core.types.enumerated.MessageSecurityMode;
import org.eclipse.milo.opcua.stack.core.types.structured.EndpointDescription;
import org.eclipse.milo.opcua.stack.core.types.structured.UserTokenPolicy;
import java.util.List;
import java.util.concurrent.CompletableFuture;
public class MiloExample {
public static void main(String[] args) throws Exception {
// 创建一个OPC UA客户端配置对象
OpcUaClientConfig config = OpcUaClientConfig.builder()
.setApplicationName("Eclipse Milo Example Client")
.setEndpoint(getEndpoint())
.setIdentityProvider(new UsernameProvider("user", "password"))
.setRequestTimeout(uint(5000))
.build();
// 创建一个OPC UA客户端
OpcUaClient client = OpcUaClient.create(config);
// 连接到OPC UA服务器
CompletableFuture<OpcUaClient> connectFuture = client.connect();
connectFuture.get();
// 读取节点的值
CompletableFuture<DataValue> readFuture = client.readValue(0, "ns=2;s=MyVariable");
DataValue value = readFuture.get();
// 输出节点的值
System.out.println("Value: " + value.getValue());
// 断开连接
CompletableFuture<Void> disconnectFuture = client.disconnect();
disconnectFuture.get();
}
private static EndpointDescription getEndpoint() {
// TODO: Replace with your OPC UA server endpoint URL
String endpointUrl = "opc.tcp://example.com:12345";
// 创建一个匿名用户令牌策略
UserTokenPolicy anonymousPolicy = UserTokenPolicy.ANONYMOUS;
// 创建一个安全策略
SecurityPolicy securityPolicy = SecurityPolicy.None;
// 创建一个消息安全模式
MessageSecurityMode messageSecurityMode = MessageSecurityMode.None;
// 创建一个终端描述对象
EndpointDescription endpoint = new EndpointDescription(
endpointUrl,
"urn:eclipse:milo:example",
null,
securityPolicy.getUri(),
messageSecurityMode,
anonymousPolicy,
null
);
return endpoint;
}
private static UnsignedInteger uint(int value) {
return new UnsignedInteger(value);
}
}
```
在这个示例中,我们首先创建了一个OPC UA客户端配置对象,并设置了应用程序名称、连接终端、身份验证提供程序和请求超时等参数。
然后我们创建了一个OPC UA客户端,并使用连接方法连接到OPC UA服务器。
接下来,我们使用readValue方法读取节点的值,并输出节点的值。
最后,我们使用disconnect方法断开连接。
请注意,在这个示例中我们使用了一个匿名用户令牌策略和安全策略为None,这是为了简化示例。在实际使用中,您需要根据您的需求选择适当的用户令牌策略和安全策略。
希望这个示例能够帮助您了解如何使用Eclipse Milo创建一个简单的OPC UA客户端。
阅读全文
相关推荐










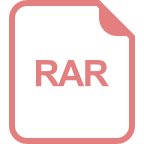







