import random # sandwich ingredients and their associated calories ingredients = { "Bread": 100, "Ham": 150, "Lettuce": 10, "Tomato": 20, "Mayonnaise": 50, "Cheese": 120 } # prompt user for calorie range min_calories = int(input("Enter minimum calories: ")) max_calories = int(input("Enter maximum calories: ")) # calculate the minimum and maximum calories for the sandwich min_sandwich_calories = 2 * ingredients["Bread"] + min(ingredients.values()) * 2 max_sandwich_calories = 2 * ingredients["Bread"] + max(ingredients.values()) * 2 # check if the calorie range is valid if max_calories < min_sandwich_calories: print("Sorry, it is impossible to create a sandwich within the given calorie range.") else: # create the sandwich sandwich = ["Bread", "Bread"] sandwich_calories = 2 * ingredients["Bread"] while sandwich_calories < min_calories: # add random ingredient ingredient = random.choice(list(ingredients.keys())) sandwich.append(ingredient) sandwich_calories += ingredients[ingredient] while sandwich_calories <= max_calories: # add random ingredient ingredient = random.choice(list(ingredients.keys())) # check if the ingredient is the same as the previous one if len(sandwich) >= 3 and ingredient == sandwich[-2]: continue sandwich.append(ingredient) sandwich_calories += ingredients[ingredient] # check if the sandwich is already at the maximum calorie limit if sandwich_calories == max_sandwich_calories: break # add the last slice of bread sandwich.append("Bread") # print the sandwich and its total calories print("Your sandwich:", sandwich) print("Total calories:", sandwich_calories)
时间: 2024-03-22 19:39:32 浏览: 13
以下是转换后的 C# 代码,您可以直接将其复制粘贴到 Visual Studio 中进行编译运行:
```
using System;
using System.Collections.Generic;
namespace SandwichCalories
{
class Program
{
static void Main(string[] args)
{
// sandwich ingredients and their associated calories
Dictionary<string, int> ingredients = new Dictionary<string, int>()
{
{ "Bread", 100 },
{ "Ham", 150 },
{ "Lettuce", 10 },
{ "Tomato", 20 },
{ "Mayonnaise", 50 },
{ "Cheese", 120 }
};
// prompt user for calorie range
Console.Write("Enter minimum calories: ");
int min_calories = int.Parse(Console.ReadLine());
Console.Write("Enter maximum calories: ");
int max_calories = int.Parse(Console.ReadLine());
// calculate the minimum and maximum calories for the sandwich
int min_sandwich_calories = 2 * ingredients["Bread"] + ingredients.Values.Min() * 2;
int max_sandwich_calories = 2 * ingredients["Bread"] + ingredients.Values.Max() * 2;
// check if the calorie range is valid
if (max_calories < min_sandwich_calories)
{
Console.WriteLine("Sorry, it is impossible to create a sandwich within the given calorie range.");
}
else
{
// create the sandwich
List<string> sandwich = new List<string> { "Bread", "Bread" };
int sandwich_calories = 2 * ingredients["Bread"];
while (sandwich_calories < min_calories)
{
// add random ingredient
string ingredient = ingredients.Keys.ElementAt(new Random().Next(ingredients.Count));
sandwich.Add(ingredient);
sandwich_calories += ingredients[ingredient];
}
while (sandwich_calories <= max_calories)
{
// add random ingredient
string ingredient = ingredients.Keys.ElementAt(new Random().Next(ingredients.Count));
// check if the ingredient is the same as the previous one
if (sandwich.Count >= 3 && ingredient == sandwich[sandwich.Count - 2])
{
continue;
}
sandwich.Add(ingredient);
sandwich_calories += ingredients[ingredient];
// check if the sandwich is already at the maximum calorie limit
if (sandwich_calories == max_sandwich_calories)
{
break;
}
}
// add the last slice of bread
sandwich.Add("Bread");
// print the sandwich and its total calories
Console.WriteLine("Your sandwich: " + string.Join(", ", sandwich));
Console.WriteLine("Total calories: " + sandwich_calories);
}
}
}
}
```
相关推荐
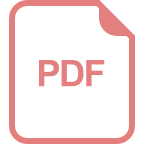
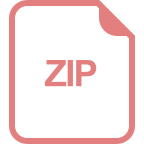
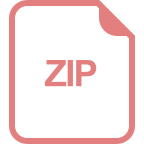














