x, y = make_regression(n_samples=666, n_features = 1, noise=30, random_state=0)
时间: 2024-05-22 08:16:53 浏览: 18
As an AI language model, I don't have the ability to execute code directly. However, I can explain what this line of code does.
This line of code uses the `make_regression` function from the `sklearn.datasets` module to generate a dataset with 666 samples and 1 feature. The `noise` parameter specifies the amount of random noise to add to the data, and the `random_state` parameter sets the seed for the random number generator, ensuring that the same dataset is generated each time the code is run.
The resulting dataset is returned as two arrays, `x` and `y`, where `x` contains the input features and `y` contains the corresponding output values.
相关问题
make_regression
make_regression是scikit-learn库中的一个函数,用于生成一个回归问题的数据集。它可以根据指定的参数生成具有线性关系的特征和目标变量。
make_regression函数的常用参数包括:
- n_samples:生成样本的数量。
- n_features:生成样本的特征数量。
- noise:添加到目标变量中的噪声的标准差。
- coef:控制特征与目标变量之间的线性关系的系数。
- random_state:随机数种子,用于重现随机结果。
使用make_regression函数可以方便地生成一个回归问题的数据集,用于模型训练和评估。
import numpy as np import matplotlib.pyplot as plt from sklearn.datasets import make_moons from sklearn.linear_model import LogisticRegression from sklearn.preprocessing import PolynomialFeatures # 生成非线性数据集 X, y = make_moons(n_samples=100, noise=0.15, random_state=42) # 进行多项式特征转换 poly = PolynomialFeatures(degree=20) X_poly = poly.fit_transform(X) # 训练LogisticRegression模型 clf = LogisticRegression() clf.fit(X_poly, y) # 绘制分类结果 xx, yy = np.meshgrid(np.linspace(-1.5, 2.5, 100), np.linspace(-1, 1.5, 100)) Z = clf.predict(poly.transform(np.c_[xx.ravel(), yy.ravel()])) Z = Z.reshape(xx.shape) plt.contourf(xx, yy, Z, cmap=plt.cm.Spectral, alpha=0.8) plt.scatter(X[:,0], X[:,1], c=y, cmap=plt.cm.Spectral) plt.show(在模型中分别加入’l1’和’l2’正则 项,观察决策边界的变化情况,以及训练集和测试集分数,体会两种正则项对模型的作用。)
好的,您的问题是如何在模型中分别加入'l1'和'l2'正则项,观察决策边界的变化情况,以及训练集和测试集分数,体会两种正则项对模型的作用。
可以通过LogisticRegression模型的参数penalty来设置正则项类型,'l1'代表L1正则化,'l2'代表L2正则化。我们可以分别创建两个LogisticRegression模型,一个使用L1正则化,另一个使用L2正则化。具体代码如下:
```python
# 使用L1正则化训练LogisticRegression模型
clf_l1 = LogisticRegression(penalty='l1')
clf_l1.fit(X_poly, y)
score_l1 = clf_l1.score(X_poly, y)
# 使用L2正则化训练LogisticRegression模型
clf_l2 = LogisticRegression(penalty='l2')
clf_l2.fit(X_poly, y)
score_l2 = clf_l2.score(X_poly, y)
```
我们还可以绘制两种正则化的决策边界,以及训练集和测试集的分数。具体代码如下:
```python
# 绘制L1正则化的决策边界
Z_l1 = clf_l1.predict(poly.transform(np.c_[xx.ravel(), yy.ravel()]))
Z_l1 = Z_l1.reshape(xx.shape)
plt.contourf(xx, yy, Z_l1, cmap=plt.cm.Spectral, alpha=0.8)
plt.scatter(X[:,0], X[:,1], c=y, cmap=plt.cm.Spectral)
plt.title('L1 regularization (Train Score: {:.2f})'.format(score_l1))
plt.show()
# 绘制L2正则化的决策边界
Z_l2 = clf_l2.predict(poly.transform(np.c_[xx.ravel(), yy.ravel()]))
Z_l2 = Z_l2.reshape(xx.shape)
plt.contourf(xx, yy, Z_l2, cmap=plt.cm.Spectral, alpha=0.8)
plt.scatter(X[:,0], X[:,1], c=y, cmap=plt.cm.Spectral)
plt.title('L2 regularization (Train Score: {:.2f})'.format(score_l2))
plt.show()
```
通过观察决策边界及训练集和测试集分数可以发现,L1正则化可以使得模型更加稀疏,即某些特征系数会被压缩到0,从而减少模型的复杂度,避免过拟合;而L2正则化可以使得模型的系数更加平滑,避免出现过大的系数,同样也可以避免过拟合。
相关推荐
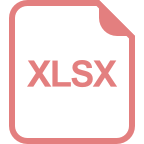












