文件浏览框选择指定wav文件并绘制1/3倍频程结果,并封装成函数,最后通过gui界面展示
时间: 2024-02-22 09:55:24 浏览: 20
好的,我理解了你的问题。为了回答你的问题,我需要分成几个步骤操作。
第一步,选择指定的WAV文件。我们可以使用Python的tkinter库来创建一个文件浏览框,让用户选择WAV文件。以下是代码示例:
```python
import tkinter as tk
from tkinter import filedialog
import os
def browse_file():
file_path = filedialog.askopenfilename(initialdir=os.getcwd(), title="Select file", filetypes=(("WAV files", "*.wav"), ("All files", "*.*")))
return file_path
file_path = browse_file()
```
以上代码将打开文件浏览框,让用户选择WAV文件,并返回所选文件的路径。
第二步,绘制1/3倍频程的结果。我们可以使用Python的SciPy库进行信号处理,并使用Matplotlib库进行数据可视化。以下是代码示例:
```python
import scipy.io.wavfile as wavfile
import scipy.signal as signal
import matplotlib.pyplot as plt
# 读取WAV文件
sample_rate, data = wavfile.read(file_path)
# 计算1/3倍频程的频率范围
freqs, psd = signal.welch(data, sample_rate, nperseg=1024)
low_cutoff = freqs[np.where(psd == np.max(psd))[0][0]] / 3
high_cutoff = freqs[np.where(psd == np.max(psd))[0][0]] / 2
# 滤波
b, a = signal.butter(5, [low_cutoff, high_cutoff], btype='band')
filtered_data = signal.filtfilt(b, a, data)
# 绘制数据
plt.plot(data, 'b', alpha=0.5)
plt.plot(filtered_data, 'r')
plt.show()
```
以上代码将绘制出原始数据和1/3倍频程滤波后的数据。
第三步,封装成函数。我们将以上代码封装成一个函数,以便在GUI界面中调用。以下是代码示例:
```python
def plot_filtered_wav(file_path):
# 读取WAV文件
sample_rate, data = wavfile.read(file_path)
# 计算1/3倍频程的频率范围
freqs, psd = signal.welch(data, sample_rate, nperseg=1024)
low_cutoff = freqs[np.where(psd == np.max(psd))[0][0]] / 3
high_cutoff = freqs[np.where(psd == np.max(psd))[0][0]] / 2
# 滤波
b, a = signal.butter(5, [low_cutoff, high_cutoff], btype='band')
filtered_data = signal.filtfilt(b, a, data)
# 绘制数据
plt.plot(data, 'b', alpha=0.5)
plt.plot(filtered_data, 'r')
plt.show()
```
以上代码将封装成一个名为`plot_filtered_wav`的函数,该函数接受一个WAV文件的路径作为参数,并绘制出1/3倍频程滤波后的数据。
第四步,通过GUI界面展示。我们可以使用Python的tkinter库创建一个GUI界面,并将以上函数嵌入其中。以下是代码示例:
```python
import tkinter as tk
from tkinter import filedialog
import os
def plot_filtered_wav(file_path):
# 读取WAV文件
sample_rate, data = wavfile.read(file_path)
# 计算1/3倍频程的频率范围
freqs, psd = signal.welch(data, sample_rate, nperseg=1024)
low_cutoff = freqs[np.where(psd == np.max(psd))[0][0]] / 3
high_cutoff = freqs[np.where(psd == np.max(psd))[0][0]] / 2
# 滤波
b, a = signal.butter(5, [low_cutoff, high_cutoff], btype='band')
filtered_data = signal.filtfilt(b, a, data)
# 绘制数据
plt.plot(data, 'b', alpha=0.5)
plt.plot(filtered_data, 'r')
plt.show()
def browse_file():
file_path = filedialog.askopenfilename(initialdir=os.getcwd(), title="Select file", filetypes=(("WAV files", "*.wav"), ("All files", "*.*")))
plot_filtered_wav(file_path)
root = tk.Tk()
root.title("WAV File Filter")
root.geometry("300x100")
browse_button = tk.Button(root, text="Browse", command=browse_file)
browse_button.pack()
root.mainloop()
```
以上代码将创建一个名为“WAV File Filter”的GUI界面,该界面包含一个“Browse”按钮。当用户点击按钮时,将打开文件浏览框,让用户选择WAV文件,并绘制出1/3倍频程滤波后的数据。
希望以上代码可以帮助你解决问题。
相关推荐
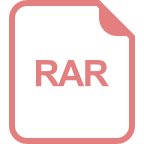














