上述问题用c语言代码解决
时间: 2023-08-31 10:06:42 浏览: 43
以下是用C语言实现的代码,其中`chessboard`数组表示棋盘,`size`表示棋盘大小,`x`和`y`表示当前处理的子棋盘左上角的坐标,`missing_x`和`missing_y`表示残缺的格子的坐标,`cover`表示当前处理的3格板的编号。
```c
#include <stdio.h>
#define MAX_SIZE 1024
int chessboard[MAX_SIZE][MAX_SIZE];
void solve(int size, int x, int y, int missing_x, int missing_y, int cover) {
if (size == 1) return;
int half = size / 2;
int center_x = x + half - 1;
int center_y = y + half - 1;
int next_cover = cover + 1;
// 左上子棋盘
if (missing_x <= center_x && missing_y <= center_y) {
chessboard[center_x][center_y+1] = chessboard[center_x+1][center_y] = chessboard[center_x+1][center_y+1] = cover;
solve(half, x, y, missing_x, missing_y, next_cover);
solve(half, x, y+half, x+half-1, y+half, next_cover);
solve(half, x+half, y, x+half, y+half-1, next_cover);
solve(half, x+half, y+half, center_x+1, center_y+1, next_cover);
}
// 右上子棋盘
else if (missing_x <= center_x && missing_y > center_y) {
chessboard[center_x][center_y] = chessboard[center_x+1][center_y] = chessboard[center_x+1][center_y+1] = cover;
solve(half, x, y, x+half-1, y+half-1, next_cover);
solve(half, x, y+half, missing_x, missing_y, next_cover);
solve(half, x+half, y, x+half, y+half-1, next_cover);
solve(half, x+half, y+half, center_x+1, center_y+1, next_cover);
}
// 左下子棋盘
else if (missing_x > center_x && missing_y <= center_y) {
chessboard[center_x][center_y] = chessboard[center_x][center_y+1] = chessboard[center_x+1][center_y+1] = cover;
solve(half, x, y, x+half-1, y+half-1, next_cover);
solve(half, x, y+half, x+half-1, y+half, next_cover);
solve(half, x+half, y, missing_x, missing_y, next_cover);
solve(half, x+half, y+half, center_x+1, center_y+1, next_cover);
}
// 右下子棋盘
else {
chessboard[center_x][center_y] = chessboard[center_x][center_y+1] = chessboard[center_x+1][center_y] = cover;
solve(half, x, y, x+half-1, y+half-1, next_cover);
solve(half, x, y+half, x+half-1, y+half, next_cover);
solve(half, x+half, y, x+half, y+half-1, next_cover);
solve(half, x+half, y+half, missing_x, missing_y, next_cover);
}
}
int main() {
int size, missing_x, missing_y;
printf("请输入棋盘大小和残缺格子的坐标:");
scanf("%d %d %d", &size, &missing_x, &missing_y);
chessboard[missing_x][missing_y] = -1;
solve(size, 0, 0, missing_x, missing_y, 0);
printf("每个格子上的编号如下:\n");
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
printf("%4d ", chessboard[i][j]);
}
printf("\n");
}
return 0;
}
```
运行程序时,输入棋盘大小和残缺格子的坐标,程序会输出每个格子上的编号,其中-1表示残缺的格子,其余的数字表示3格板的编号。
相关推荐
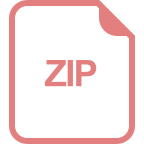
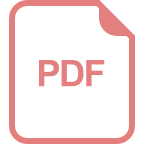
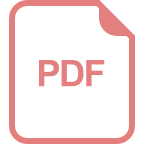














