用Python实现具体的hough变换
时间: 2023-11-05 15:03:12 浏览: 93
以下是一个Python实现的Hough变换的示例代码。这里的实现是用来检测图像中的直线。
```python
import numpy as np
import cv2
def hough_lines(img, rho, theta, threshold):
"""
返回一组检测到的直线
"""
# 初始化一个空的投票矩阵
height, width = img.shape
diag_len = int(np.ceil(np.sqrt(height**2 + width**2))) # 对角线长度
accumulator = np.zeros((2 * diag_len, 180), dtype=np.uint8)
# 取得图像中所有非零像素的坐标
coords = np.column_stack(np.where(img > 0))
# 对于每个非零像素,计算它们在Hough空间中的所有可能的直线
for x, y in coords:
for t in range(0, 180):
r = int((x * np.cos(np.deg2rad(t))) + (y * np.sin(np.deg2rad(t))))
accumulator[r + diag_len, t] += 1
# 返回所有投票数超过阈值的直线
lines = []
for r, theta in np.argwhere(accumulator >= threshold):
lines.append((r - diag_len, theta))
return lines
# 加载图像并将其转换为灰度
image = cv2.imread('image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 对灰度图像应用Canny边缘检测器
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# 运行Hough变换检测直线
lines = hough_lines(edges, 1, np.pi / 180, 200)
# 将检测到的直线绘制到原始图像上
for r, theta in lines:
a = np.cos(theta)
b = np.sin(theta)
x0 = a * r
y0 = b * r
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
cv2.line(image, (x1, y1), (x2, y2), (0, 0, 255), 2)
# 显示结果
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个示例代码加载了一个名为`image.jpg`的图像,并将其转换为灰度图像。然后,它使用Canny边缘检测器检测边缘,并通过调用`hough_lines`函数运行Hough变换来检测直线。最后,它将检测到的直线绘制到原始图像上,并显示结果。
阅读全文
相关推荐
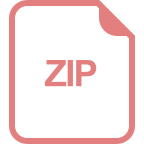



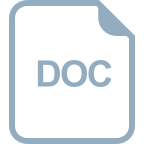







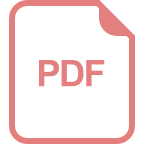



