定义类X、Y、Z,函数h(X *),满足:类X有私有成员i,Y的成员函数g(X *) 是X的友元函数,实现对X的成员i加1,类Z是类X的友元类,其成员函数f(X *)实现对X的成员i加5,函数h(X *)是X的友元函数,实现对X的成员i加10。在一个文件中声明类,在一个文件中实现类,在另一个文件中测试类
时间: 2023-06-13 16:07:43 浏览: 102
以下是类X、Y、Z的声明和函数h的声明:
**X.h:**
```c++
#ifndef X_H
#define X_H
class Y; // 前置声明
class X {
private:
int i;
public:
X(int i = 0);
friend void h(X* x);
friend class Z;
friend void Y::g(X* x); // 友元函数的声明可以在类外定义
// 友元函数的定义必须在类外
friend void setX(X* x, int i);
};
#endif
```
**Y.h:**
```c++
#ifndef Y_H
#define Y_H
#include "X.h"
class Y {
public:
void g(X* x);
};
#endif
```
**Z.h:**
```c++
#ifndef Z_H
#define Z_H
#include "X.h"
class Z {
public:
void f(X* x);
};
#endif
```
**h.h:**
```c++
#ifndef H_H
#define H_H
#include "X.h"
void h(X* x);
#endif
```
接下来是类的实现:
**X.cpp:**
```c++
#include "X.h"
X::X(int i) : i(i) {}
void setX(X* x, int i) {
x->i = i;
}
```
**Y.cpp:**
```c++
#include "Y.h"
#include "X.h"
void Y::g(X* x) {
x->i += 1;
}
```
**Z.cpp:**
```c++
#include "Z.h"
#include "X.h"
void Z::f(X* x) {
x->i += 5;
}
```
最后是测试类的文件:
**main.cpp:**
```c++
#include <iostream>
#include "X.h"
#include "Y.h"
#include "Z.h"
#include "h.h"
using namespace std;
int main() {
X x(0);
Y y;
Z z;
cout << x.i << endl; // 0
y.g(&x);
cout << x.i << endl; // 1
z.f(&x);
cout << x.i << endl; // 6
h(&x);
cout << x.i << endl; // 16
return 0;
}
```
注意,函数h的定义需要访问X的私有成员i,因此需要将其声明为X的友元函数。函数h的定义可以在类外实现,也可以在类内实现。
相关推荐
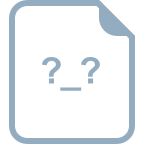
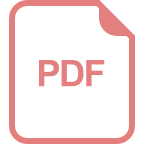
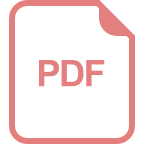















