Java实现实现最长公共子序列
时间: 2024-05-10 07:16:19 浏览: 56
最长公共子序列(Longest Common Subsequence,LCS)指的是两个字符串中最长的公共子序列,可以不连续。Java 实现最长公共子序列可以使用动态规划算法,具体实现如下:
```java
public static String LCS(String str1, String str2) {
int m = str1.length();
int n = str2.length();
int[][] dp = new int[m + 1][n + 1];
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (str1.charAt(i - 1) == str2.charAt(j - 1)) {
dp[i][j] = dp[i - 1][j - 1] + 1;
} else {
dp[i][j] = Math.max(dp[i - 1][j], dp[i][j - 1]);
}
}
}
int index = dp[m][n];
char[] lcs = new char[index];
int i = m, j = n;
while (i > 0 && j > 0) {
if (str1.charAt(i - 1) == str2.charAt(j - 1)) {
lcs[--index] = str1.charAt(i - 1);
i--;
j--;
} else if (dp[i - 1][j] > dp[i][j - 1]) {
i--;
} else {
j--;
}
}
return new String(lcs);
}
```
在上面的代码中,我们使用一个二维数组 dp 来存储最长公共子序列的长度。dp[i][j] 表示 str1 中前 i 个字符和 str2 中前 j 个字符的最长公共子序列长度。对于两个字符相等的情况,dp[i][j] 等于 dp[i-1][j-1] + 1,否则为 Math.max(dp[i-1][j], dp[i][j-1])。最后,我们根据 dp 数组构造最长公共子序列。
阅读全文
相关推荐
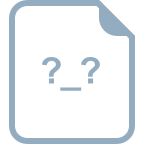


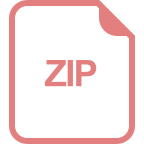
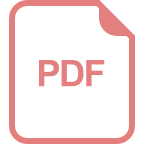
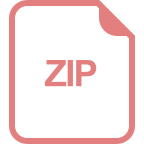
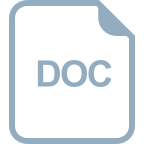
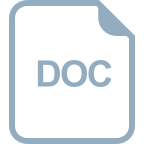
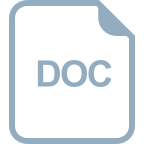
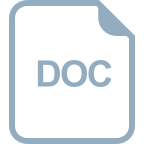





