android rgb图像byte[] 转 Bitmap
时间: 2023-12-09 07:05:39 浏览: 92
可以使用以下代码将一个 RGB 图像的 byte[] 数组转换为 Bitmap:
```java
// 定义图片宽高和颜色模式
int width = 640;
int height = 480;
Bitmap bitmap = Bitmap.createBitmap(width, height, Bitmap.Config.RGB_565);
// 将 byte[] 数组转换为 Bitmap
byte[] imageBytes = ... ; // RGB 图像的 byte[] 数组
ByteBuffer buffer = ByteBuffer.wrap(imageBytes);
bitmap.copyPixelsFromBuffer(buffer);
```
在上面的代码中,我们首先定义了图片的宽高和颜色模式,然后创建一个 Bitmap 对象。接下来,我们将 byte[] 数组转换为 ByteBuffer,并使用 `copyPixelsFromBuffer()` 方法将 ByteBuffer 中的数据拷贝到 Bitmap 对象中。最终得到的 Bitmap 对象就是我们需要的 RGB 图像。
相关问题
android nv21 buffer 转bitmap
可以使用以下代码将NV21格式的数据转换为Bitmap:
```java
public static Bitmap nv21ToBitmap(byte[] data, int width, int height) {
YuvImage yuvImage = new YuvImage(data, ImageFormat.NV21, width, height, null);
ByteArrayOutputStream stream = new ByteArrayOutputStream();
yuvImage.compressToJpeg(new Rect(0, 0, width, height), 100, stream);
byte[] jpegData = stream.toByteArray();
BitmapFactory.Options options = new BitmapFactory.Options();
options.inPreferredConfig = Bitmap.Config.RGB_565; // 根据实际情况选择Bitmap.Config
Bitmap bitmap = BitmapFactory.decodeByteArray(jpegData, 0, jpegData.length, options);
return bitmap;
}
```
其中,`data` 是NV21格式的数据,`width` 和 `height` 分别是图像的宽度和高度。函数将NV21格式的数据先转换为JPEG格式的数据,然后再通过`BitmapFactory`将JPEG数据转换为Bitmap。
Android nv21 转bitmap
在Android开发中,NV21是YUV420SP格式的一种,这是一种常用的图像数据格式,特别是在Android的Camera API中。NV21格式的数据并不直接适合在UI中显示,因此通常需要将其转换为Bitmap对象。以下是将NV21格式的数据转换为Bitmap的一个基本步骤:
1. 获取NV21数据缓冲区的字节数组,这个通常从Camera的回调中获取。
2. 使用`MediaCodec`或`YuvImage`类来处理YUV数据。
3. 将处理后的数据转换为Bitmap。
以下是一个简化的代码示例,使用`YuvImage`类将NV21数据转换为Bitmap:
```java
// 假设byte[] nv21Data是从Camera回调中获取到的NV21格式的字节数组,width和height是图像的宽度和高度
byte[] nv21Data = ...;
int width = ...;
int height = ...;
// 创建一个YuvImage对象
YuvImage yuvImage = new YuvImage(nv21Data, ImageFormat.NV21, width, height, null);
// 用于存储转换后的RGB数据
ByteArrayOutputStream out = new ByteArrayOutputStream();
// 将YUV数据转换为JPEG格式的字节数组
yuvImage.compressToJpeg(new Rect(0, 0, width, height), 100, out);
// 将JPEG字节数组转换为Bitmap
Bitmap bmp = BitmapFactory.decodeByteArray(out.toByteArray(), 0, out.size());
// 使用bmp对象进行后续操作...
```
在实际应用中,你可能需要根据实际需求调整压缩质量参数以及处理图像尺寸等。
阅读全文
相关推荐
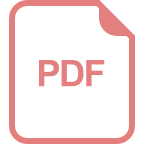
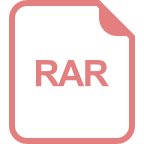
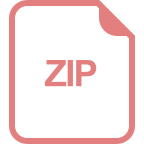









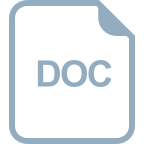
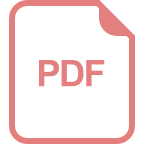
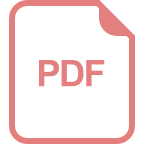
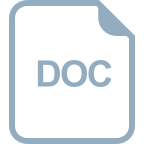
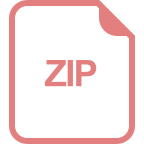