pytorch 不改变原模型情况下,替换 forward 中的torch.gather操作
时间: 2023-09-05 15:05:39 浏览: 155
### 回答1:
我可以提供一些建议,但无法保证它可以在没有改变原模型的情况下替换 torch.gather 操作。首先,可以尝试使用 torch.index_select 来实现同样的功能。其次,可以考虑使用 torch.masked_select 来替换 torch.gather,具体可以参考 PyTorch 的文档。
### 回答2:
在PyTorch中,如果想要在不改变原模型的情况下替换forward函数中的torch.gather操作,可以使用torch.index_select函数来实现相同的功能。torch.index_select函数接受一个tensor和一个维度索引作为参数,返回按照指定维度索引的元素。
首先,我们需要理解torch.gather操作的作用。torch.gather可以按照指定的维度,在一个tensor中进行索引,并返回相应的值。例如,对于一个大小为(3, 4)的tensor,我们可以通过torch.gather(tensor, 0, index)来按照第0个维度的索引index来获取对应值。
下面是一个示例代码,展示如何使用torch.index_select替换forward函数中的torch.gather操作:
```python
import torch
import torch.nn as nn
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.weights = nn.Parameter(torch.randn(3, 4))
def forward(self, index):
# 使用torch.gather操作
output = torch.gather(self.weights, 0, index)
return output
def replace_forward(self, index):
# 使用torch.index_select替换torch.gather操作
output = torch.index_select(self.weights, 0, index)
return output
```
在上面的示例代码中,MyModel类的forward函数中使用了torch.gather操作,而replace_forward函数中则使用了torch.index_select来实现相同的功能。这样,我们可以在不改变原模型的情况下替换forward函数中的torch.gather操作。
### 回答3:
在不改变原模型的情况下,我们可以通过使用其他的操作来替换`torch.gather`。
`torch.gather`操作通常用于根据索引从输入的张量中提取特定元素。它的一般形式是`torch.gather(input, dim, index, out=None)`,其中`input`是输入张量,`dim`是提取索引的维度,`index`是包含提取索引的张量。
为了替换`torch.gather`操作,我们可以使用`torch.index_select`和`torch.unsqueeze`来实现相似的功能。
首先,我们可以使用`torch.index_select`操作来选择指定维度上的索引。这个操作的一般形式是`torch.index_select(input, dim, index, out=None)`,其中`input`是要选择的张量,`dim`是选择的维度,`index`是包含索引的一维张量。
然后,我们可以使用`torch.unsqueeze`操作来在选择的维度上增加一个维度。这个操作的一般形式是`torch.unsqueeze(input, dim, out=None)`,其中`input`是要增加维度的张量,`dim`是要增加的维度。
综上所述,为了替换`torch.gather`操作,我们可以使用以下代码:
```python
import torch
class Model(torch.nn.Module):
def __init__(self):
super(Model, self).__init__()
def forward(self, input, index):
# 替换 torch.gather 的操作
output = torch.index_select(input, 1, index.unsqueeze(1)).squeeze(1)
return output
```
在上面的代码中,我们使用`torch.index_select`选择了指定维度`dim=1`上的索引,并使用`torch.unsqueeze`增加了一个维度。最后,我们使用`squeeze`操作将这个额外的维度去除,以匹配`torch.gather`操作的输出。
这样,我们就在不改变原模型的情况下替换了`torch.gather`操作,实现了相似的功能。
相关推荐
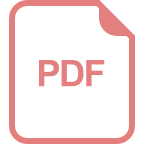
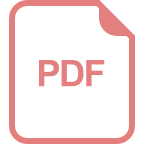
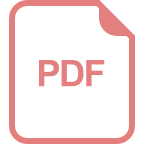














