def forward(self, x): xyz = x.permute(0, 2, 1) batch_size, _, _ = x.size() # B, D, N x = F.relu(self.bn1(self.conv1(x))) # B, D, N x = F.relu(self.bn2(self.conv2(x))) x = x.permute(0, 2, 1) new_xyz, new_feature = sample_and_group(npoint=512, radius=0.15, nsample=32, xyz=xyz, points=x) feature_0 = self.gather_local_0(new_feature) feature = feature_0.permute(0, 2, 1) new_xyz, new_feature = sample_and_group(npoint=256, radius=0.2, nsample=32, xyz=new_xyz, points=feature) feature_1 = self.gather_local_1(new_feature) x = self.pt_last(feature_1) x = torch.cat([x, feature_1], dim=1) x = self.conv_fuse(x) x = F.adaptive_max_pool1d(x, 1).view(batch_size, -1) x = F.leaky_relu(self.bn6(self.linear1(x)), negative_slope=0.2) x = self.dp1(x) x = F.leaky_relu(self.bn7(self.linear2(x)), negative_slope=0.2) x = self.dp2(x) x = self.linear3(x) return x
时间: 2024-01-27 22:04:02 浏览: 28
这段代码是一个PyTorch的神经网络模型的前向传播函数,用于对输入x做推理得到输出结果。该模型为PointNet++,用于处理点云数据。该函数的输入为点云数据x,输出为该点云数据的特征向量表示。具体实现过程中,该模型对点云数据进行了一系列处理,包括对点云数据进行卷积、池化等操作,最终得到点云数据的特征向量表示。
相关问题
class CNN(nn.Module): def __init__(self,input_size,output_size): super(CNN, self).__init__() self.B = B self.relu = nn.ReLU(inplace=True) self.conv1 = nn.Sequential( nn.Conv1d(in_channels=input_size, out_channels=64, kernel_size=2), # 24 - 2 + 1 = 23 nn.ReLU(), nn.MaxPool1d(kernel_size=2, stride=1), # 23 - 2 + 1 = 22 ) self.conv2 = nn.Sequential( nn.Conv1d(in_channels=64, out_channels=128, kernel_size=2), # 22 - 2 + 1 = 21 nn.ReLU(), nn.MaxPool2d(kernel_size=2, stride=1), # 21 - 2 + 1 = 20 ) self.Linear1 = nn.Linear(self.B * 127 * 20, self.B * 50) self.Linear2 = nn.Linear(self.B * 50 , output_size) def forward(self, x): # [batch_size, n_features, data_len] x = x.permute(0, 2, 1) x = self.conv1(x) x = self.conv2(x) x = x.view(-1) x = self.Linear1(x) x = self.relu(x) x = self.Linear2(x) x = x.view(x.shape[0], -1) return x
这段代码定义了一个CNN模型,它包含了两个卷积层和两个全连接层。下面是对代码的解释:
```python
class CNN(nn.Module):
def __init__(self, input_size, output_size):
super(CNN, self).__init__()
self.B = B
self.relu = nn.ReLU(inplace=True)
self.conv1 = nn.Sequential(
nn.Conv1d(in_channels=input_size, out_channels=64, kernel_size=2), # 24 - 2 + 1 = 23
nn.ReLU(),
nn.MaxPool1d(kernel_size=2, stride=1), # 23 - 2 + 1 = 22
)
self.conv2 = nn.Sequential(
nn.Conv1d(in_channels=64, out_channels=128, kernel_size=2), # 22 - 2 + 1 = 21
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=1), # 21 - 2 + 1 = 20
)
self.Linear1 = nn.Linear(self.B * 127 * 20, self.B * 50)
self.Linear2 = nn.Linear(self.B * 50 , output_size)
def forward(self, x):
# [batch_size, n_features, data_len]
x = x.permute(0, 2, 1)
x = self.conv1(x)
x = self.conv2(x)
x = x.view(-1)
x = self.Linear1(x)
x = self.relu(x)
x = self.Linear2(x)
x = x.view(x.shape[0], -1)
return x
```
在构造函数`__init__`中,模型初始化了一些参数并定义了网络的各个层。其中,`self.conv1`是一个包含了一个卷积层、ReLU激活函数和最大池化层的序列。`self.conv2`也是一个类似的序列。`self.Linear1`和`self.Linear2`分别是两个全连接层。
在前向传播函数`forward`中,输入数据首先进行形状变换,然后通过卷积层和激活函数进行特征提取和降维。之后,将特征展平并通过全连接层进行预测。最后,输出结果进行形状变换以匹配预期的输出形状。
需要注意的是,代码中的一些变量(如`B`)没有给出具体的定义,你可能需要根据自己的需求进行修改。
希望这个解释对你有所帮助!如果还有其他问题,请随时提问。
class BayarConv2d(nn.Module): def __init__(self, in_channels, out_channels, kernel_size=5, stride=1, padding=0): self.in_channels = in_channels self.out_channels = out_channels self.kernel_size = kernel_size self.stride = stride self.padding = padding self.minus1 = (torch.ones(self.in_channels, self.out_channels, 1) * -1.000) super(BayarConv2d, self).__init__() # only (kernel_size ** 2 - 1) trainable params as the center element is always -1 self.kernel = nn.Parameter(torch.rand(self.in_channels, self.out_channels, kernel_size ** 2 - 1), requires_grad=True) def bayarConstraint(self): self.kernel.data = self.kernel.permute(2, 0, 1) self.kernel.data = torch.div(self.kernel.data, self.kernel.data.sum(0)) self.kernel.data = self.kernel.permute(1, 2, 0) ctr = self.kernel_size ** 2 // 2 real_kernel = torch.cat((self.kernel[:, :, :ctr], self.minus1.to(self.kernel.device), self.kernel[:, :, ctr:]), dim=2) real_kernel = real_kernel.reshape((self.out_channels, self.in_channels, self.kernel_size, self.kernel_size)) return real_kernel def forward(self, x): x = F.conv2d(x, self.bayarConstraint(), stride=self.stride, padding=self.padding) return x这个类最后得到了什么
这个类实现了一个卷积神经网络层,其中使用了贝叶斯方法对卷积核进行约束,使其满足一定的先验分布。
通过类的初始化函数,用户可以指定输入通道数、输出通道数、卷积核大小、卷积步长和填充大小等参数。
在类的初始化函数中,首先通过类的成员变量存储输入通道数、输出通道数、卷积核大小、卷积步长和填充大小等参数。然后,使用 PyTorch 的 nn.Parameter 函数创建一个可训练的卷积核张量 self.kernel。这个张量的大小为 (in_channels, out_channels, kernel_size ** 2 - 1),其中 kernel_size ** 2 - 1 表示卷积核中除去中心位置的参数数量,因为中心位置的参数总是固定为 -1。
在 BayarConv2d 类中,还定义了一个名为 bayarConstraint 的函数,用于对卷积核进行约束。在这个函数中,首先将 self.kernel 的维度转换为 (kernel_size ** 2 - 1, in_channels, out_channels),然后对 self.kernel 的第一个维度进行归一化,使得每个卷积核的参数总和为 1。接着,将 self.kernel 的维度再次转换为 (in_channels, out_channels, kernel_size ** 2 - 1),然后使用 torch.cat() 函数将中心位置的 -1 插入到 self.kernel 的第三个维度的中心位置,得到最终的约束卷积核 real_kernel。最后,将 real_kernel 的维度转换为 (out_channels, in_channels, kernel_size, kernel_size)。
最后,定义了 forward 函数,实现了 BayarConv2d 类的前向传播过程。在 forward 函数中,使用 PyTorch 的 F.conv2d() 函数对输入进行卷积操作,其中卷积核为调用 bayarConstraint 函数得到的约束卷积核 real_kernel。
相关推荐
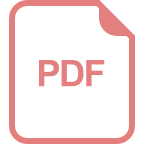
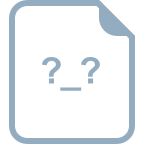












