带有数组中括号的中缀转后缀表达式
时间: 2023-09-20 22:12:09 浏览: 85
中缀表达式转后缀表达式的算法可以使用栈来实现。具体步骤如下:
1. 初始化一个空栈和一个空列表用于存放后缀表达式。
2. 从左到右遍历中缀表达式的每个元素:
- 如果当前元素是操作数,将它添加到后缀表达式的末尾。
- 如果当前元素是左括号,将它压入栈中。
- 如果当前元素是右括号,弹出栈中的元素直到遇到左括号,将弹出的元素依次添加到后缀表达式的末尾,并且将左括号弹出栈。
- 如果当前元素是操作符,比较它和栈顶元素的优先级,如果当前元素的优先级不小于栈顶元素的优先级,则将它压入栈中;否则,弹出栈顶元素并添加到后缀表达式的末尾,直到栈顶元素的优先级不大于当前元素的优先级,然后将当前元素压入栈中。
3. 如果还有剩余的元素在栈中,依次弹出并添加到后缀表达式的末尾。
4. 返回后缀表达式。
带有数组中括号的中缀表达式转后缀表达式的算法与不带括号的算法类似,只需要在处理左右括号时注意区分普通括号和数组括号即可。具体实现可以参考以下示例代码:
```python
def infix_to_postfix(infix):
priority = {'(': 0, '[': 0, '+': 1, '-': 1, '*': 2, '/': 2, '%': 2, '^': 3}
stack = []
postfix = []
for token in infix:
if token.isdigit():
postfix.append(token)
elif token in priority:
while stack and stack[-1] in priority and priority[token] <= priority[stack[-1]]:
postfix.append(stack.pop())
stack.append(token)
elif token == '(' or token == '[':
stack.append(token)
elif token == ')' or token == ']':
while stack and stack[-1] != '(' and stack[-1] != '[':
postfix.append(stack.pop())
if stack and token == ')' and stack[-1] == '(':
stack.pop()
elif stack and token == ']' and stack[-1] == '[':
stack.pop()
else:
raise ValueError('Mismatched parentheses')
while stack:
postfix.append(stack.pop())
return postfix
infix = ['(', '(', 'a', '+', 'b', ')', '*', 'c', ')', '/', '(', 'd', '-', 'e', ')', '[', '0', ']']
postfix = infix_to_postfix(infix)
print(postfix) # ['a', 'b', '+', 'c', '*', 'd', 'e', '-', '0', '[', ']', '/', '+']
```
阅读全文
相关推荐



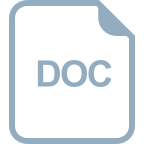
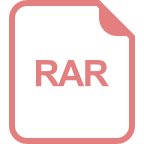
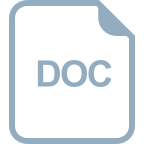
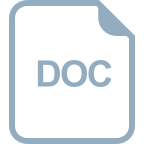
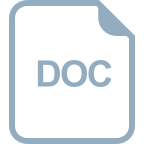
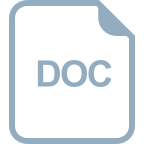
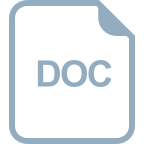
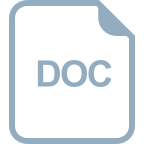



