带有数组中括号的中缀转后缀
时间: 2023-06-30 14:24:16 浏览: 48
如果中缀表达式中包含数组下标,例如 `a[2]+3*4-(b[1]+c[0])*d[2]`,则需要稍微修改中缀转后缀算法。
具体来说,我们可以将数组下标视为操作数的一部分,例如将 `a[2]` 视为一个整体。在扫描中缀表达式时,需要将数组下标作为一个整体进行处理,并将其输出到后缀表达式中。下面是一个简单的 C++ 实现示例:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 判断是否为运算符
bool isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
// 判断运算符优先级
int getPriority(char c) {
if (c == '+' || c == '-') {
return 1;
} else if (c == '*' || c == '/') {
return 2;
} else {
return 0;
}
}
// 中缀转后缀
string infixToPostfix(string infix) {
stack<char> s; // 运算符栈
string postfix; // 后缀表达式
for (int i = 0; i < infix.length(); i++) {
char c = infix[i];
// 如果是操作数或数组下标,直接输出
if (isdigit(c) || isalpha(c)) {
postfix += c;
} else if (c == '[') { // 如果是左中括号
// 将数组名输出
postfix += c;
i++;
while (i < infix.length() && infix[i] != ']') {
postfix += infix[i];
i++;
}
postfix += infix[i];
} else if (isOperator(c)) { // 如果是运算符
// 弹出优先级大于等于该运算符的所有运算符
while (!s.empty() && getPriority(s.top()) >= getPriority(c)) {
postfix += s.top();
s.pop();
}
// 将该运算符压入栈中
s.push(c);
} else if (c == '(') { // 如果是左括号
s.push(c);
} else if (c == ')') { // 如果是右括号
// 弹出左括号之后的所有运算符
while (!s.empty() && s.top() != '(') {
postfix += s.top();
s.pop();
}
// 弹出左括号
if (!s.empty() && s.top() == '(') {
s.pop();
}
}
}
// 将栈中剩余的运算符弹出并输出
while (!s.empty()) {
postfix += s.top();
s.pop();
}
return postfix;
}
int main() {
string infix = "a[2]+3*4-(b[1]+c[0])*d[2]"; // 中缀表达式
string postfix = infixToPostfix(infix); // 后缀表达式
cout << postfix << endl; // 输出后缀表达式
return 0;
}
```
该程序输出的结果为:`a2[+]+34*+b1c0[+]d2*+-`。
相关推荐
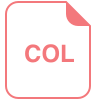













