C语言 中缀转后缀表达式,并且带有数组中括号符号
时间: 2023-10-29 07:17:02 浏览: 60
下面是一个C语言实现中缀表达式转后缀表达式的示例代码,其中包含对数组中括号符号的处理:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
char stack[MAX_SIZE];
int top = -1;
// 判断是否为操作符
int isOperator(char c) {
if (c == '+' || c == '-' || c == '*' || c == '/' || c == '%' || c == '^')
return 1;
return 0;
}
// 判断优先级
int precedence(char c) {
switch (c) {
case '+':
case '-':
return 1;
case '*':
case '/':
case '%':
return 2;
case '^':
return 3;
default:
return 0;
}
}
// 将中缀表达式转换为后缀表达式
void infixToPostfix(char* infix, char* postfix) {
int i, j, len = strlen(infix);
for (i = 0, j = 0; i < len; i++) {
if (infix[i] == '(') {
stack[++top] = infix[i];
} else if (infix[i] == ')') {
while (top >= 0 && stack[top] != '(') {
postfix[j++] = stack[top--];
}
top--; // 弹出左括号
} else if (isOperator(infix[i])) {
while (top >= 0 && precedence(stack[top]) >= precedence(infix[i])) {
postfix[j++] = stack[top--];
}
stack[++top] = infix[i];
} else if (infix[i] >= '0' && infix[i] <= '9') {
postfix[j++] = infix[i];
} else if (infix[i] == '[') {
postfix[j++] = infix[i];
i++; // 跳过下一个字符,即数组下标的第一个字符
while (infix[i] != ']') {
postfix[j++] = infix[i++];
}
postfix[j++] = infix[i];
}
}
while (top >= 0) {
postfix[j++] = stack[top--];
}
postfix[j] = '\0';
}
int main() {
char infix[MAX_SIZE], postfix[MAX_SIZE];
printf("Enter an infix expression: ");
scanf("%s", infix);
infixToPostfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
return 0;
}
```
在上面的代码中,对于数组中括号符号`[]`的处理,先将左中括号`[`加入输出字符串中,然后跳过下一个字符,即数组下标的第一个字符,将数组下标中的所有字符加入输出字符串中,最后将右中括号`]`加入输出字符串中。
相关推荐
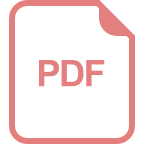
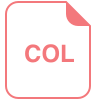













